diff options
-rw-r--r-- | .gitignore | 1 | ||||
-rw-r--r-- | python-TestSlide.spec | 390 | ||||
-rw-r--r-- | sources | 1 |
3 files changed, 392 insertions, 0 deletions
@@ -0,0 +1 @@ +/TestSlide-2.7.0.tar.gz diff --git a/python-TestSlide.spec b/python-TestSlide.spec new file mode 100644 index 0000000..6573c2f --- /dev/null +++ b/python-TestSlide.spec @@ -0,0 +1,390 @@ +%global _empty_manifest_terminate_build 0 +Name: python-TestSlide +Version: 2.7.0 +Release: 1 +Summary: A test framework for Python that makes mocking and iterating over code with tests a breeze +License: MIT +URL: https://github.com/facebook/TestSlide +Source0: https://mirrors.nju.edu.cn/pypi/web/packages/48/3c/91629f7beaa8ee2eb80c05993907cfdf859c00c1585b75d9d7e8afd09d9b/TestSlide-2.7.0.tar.gz +BuildArch: noarch + + +%description + + +[](https://github.com/facebook/TestSlide/actions?query=workflow%3ACI) +[](https://coveralls.io/github/facebook/TestSlide?branch=main) +[](https://testslide.readthedocs.io/en/main/?badge=main) +[](LICENSE) +[](https://badge.fury.io/py/TestSlide) +[](https://github.com/ambv/black) + +A test framework for Python that enable [unit testing](https://docs.python.org/3/library/unittest.html) / [TDD](https://en.wikipedia.org/wiki/Test-driven_development) / [BDD](https://en.wikipedia.org/wiki/Behavior-driven_development) to be productive and enjoyable. + +Its well behaved mocks with thorough API validations catches bugs both when code is first written or long in the future when it is changed. + +The flexibility of using them with existing `unittest.TestCase` or TestSlide's own test runner let users get its benefits without requiring refactoring existing code. + +## Quickstart + +Install: + +``` +pip install TestSlide +``` + +Scaffold the code you want to test `backup.py`: + +```python +class Backup: + def delete(self, path): + pass +``` + +Write a test case `backup_test.py` describing the expected behavior: + +```python +import testslide, backup, storage + +class TestBackupDelete(testslide.TestCase): + def setUp(self): + super().setUp() + self.storage_mock = testslide.StrictMock(storage.Client) + # Makes storage.Client(timeout=60) return self.storage_mock + self.mock_constructor(storage, 'Client')\ + .for_call(timeout=60)\ + .to_return_value(self.storage_mock) + + def test_delete_from_storage(self): + # Set behavior and assertion for the call at the mock + self.mock_callable(self.storage_mock, 'delete')\ + .for_call('/file/to/delete')\ + .to_return_value(True)\ + .and_assert_called_once() + backup.Backup().delete('/file/to/delete') +``` + +TestSlide's `StrictMock`, `mock_constructor()` and `mock_callable()` are seamlessly integrated with Python's TestCase. + +Run the test and see the failure: + +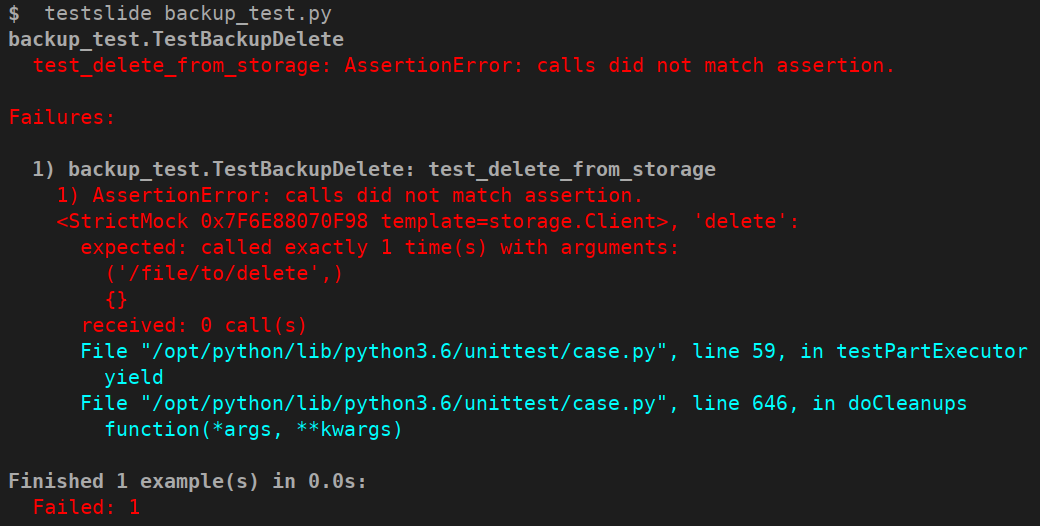 + +TestSlide's mocks failure messages guide you towards the solution, that you can now implement: + +```python +import storage + +class Backup: + def __init__(self): + self.storage = storage.Client(timeout=60) + + def delete(self, path): + self.storage.delete(path) +``` + +And watch the test go green: + +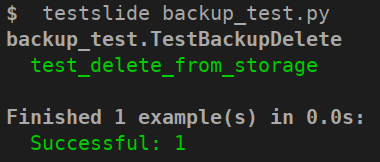 + +It is all about letting the failure messages guide you towards the solution. There's a plethora of validation inside TestSlide's mocks, so you can trust they will help you iterate quickly when writing code and also cover you when breaking changes are introduced. + +## Full documentation + +There's a lot more that TestSlide can offer, please check the full documentation at https://testslide.readthedocs.io/ to learn more. + +## Requirements + +* Linux +* Python 3 + +## Join the TestSlide community + +TestSlide is open source software, contributions are very welcome! + +See the [CONTRIBUTING](CONTRIBUTING.md) file for how to help out. + +## License + +TestSlide is MIT licensed, as found in the [LICENSE](LICENSE) file. + + +## Terms of Use + +https://opensource.facebook.com/legal/terms + + +## Privacy Policy + +https://opensource.facebook.com/legal/privacy + +%package -n python3-TestSlide +Summary: A test framework for Python that makes mocking and iterating over code with tests a breeze +Provides: python-TestSlide +BuildRequires: python3-devel +BuildRequires: python3-setuptools +BuildRequires: python3-pip +%description -n python3-TestSlide + + +[](https://github.com/facebook/TestSlide/actions?query=workflow%3ACI) +[](https://coveralls.io/github/facebook/TestSlide?branch=main) +[](https://testslide.readthedocs.io/en/main/?badge=main) +[](LICENSE) +[](https://badge.fury.io/py/TestSlide) +[](https://github.com/ambv/black) + +A test framework for Python that enable [unit testing](https://docs.python.org/3/library/unittest.html) / [TDD](https://en.wikipedia.org/wiki/Test-driven_development) / [BDD](https://en.wikipedia.org/wiki/Behavior-driven_development) to be productive and enjoyable. + +Its well behaved mocks with thorough API validations catches bugs both when code is first written or long in the future when it is changed. + +The flexibility of using them with existing `unittest.TestCase` or TestSlide's own test runner let users get its benefits without requiring refactoring existing code. + +## Quickstart + +Install: + +``` +pip install TestSlide +``` + +Scaffold the code you want to test `backup.py`: + +```python +class Backup: + def delete(self, path): + pass +``` + +Write a test case `backup_test.py` describing the expected behavior: + +```python +import testslide, backup, storage + +class TestBackupDelete(testslide.TestCase): + def setUp(self): + super().setUp() + self.storage_mock = testslide.StrictMock(storage.Client) + # Makes storage.Client(timeout=60) return self.storage_mock + self.mock_constructor(storage, 'Client')\ + .for_call(timeout=60)\ + .to_return_value(self.storage_mock) + + def test_delete_from_storage(self): + # Set behavior and assertion for the call at the mock + self.mock_callable(self.storage_mock, 'delete')\ + .for_call('/file/to/delete')\ + .to_return_value(True)\ + .and_assert_called_once() + backup.Backup().delete('/file/to/delete') +``` + +TestSlide's `StrictMock`, `mock_constructor()` and `mock_callable()` are seamlessly integrated with Python's TestCase. + +Run the test and see the failure: + +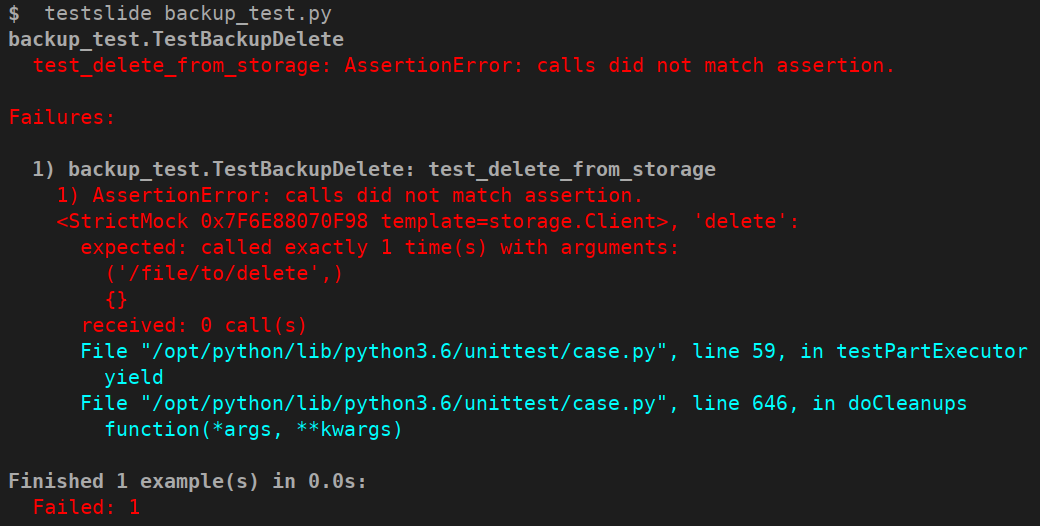 + +TestSlide's mocks failure messages guide you towards the solution, that you can now implement: + +```python +import storage + +class Backup: + def __init__(self): + self.storage = storage.Client(timeout=60) + + def delete(self, path): + self.storage.delete(path) +``` + +And watch the test go green: + +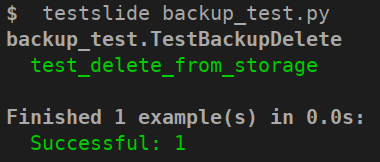 + +It is all about letting the failure messages guide you towards the solution. There's a plethora of validation inside TestSlide's mocks, so you can trust they will help you iterate quickly when writing code and also cover you when breaking changes are introduced. + +## Full documentation + +There's a lot more that TestSlide can offer, please check the full documentation at https://testslide.readthedocs.io/ to learn more. + +## Requirements + +* Linux +* Python 3 + +## Join the TestSlide community + +TestSlide is open source software, contributions are very welcome! + +See the [CONTRIBUTING](CONTRIBUTING.md) file for how to help out. + +## License + +TestSlide is MIT licensed, as found in the [LICENSE](LICENSE) file. + + +## Terms of Use + +https://opensource.facebook.com/legal/terms + + +## Privacy Policy + +https://opensource.facebook.com/legal/privacy + +%package help +Summary: Development documents and examples for TestSlide +Provides: python3-TestSlide-doc +%description help + + +[](https://github.com/facebook/TestSlide/actions?query=workflow%3ACI) +[](https://coveralls.io/github/facebook/TestSlide?branch=main) +[](https://testslide.readthedocs.io/en/main/?badge=main) +[](LICENSE) +[](https://badge.fury.io/py/TestSlide) +[](https://github.com/ambv/black) + +A test framework for Python that enable [unit testing](https://docs.python.org/3/library/unittest.html) / [TDD](https://en.wikipedia.org/wiki/Test-driven_development) / [BDD](https://en.wikipedia.org/wiki/Behavior-driven_development) to be productive and enjoyable. + +Its well behaved mocks with thorough API validations catches bugs both when code is first written or long in the future when it is changed. + +The flexibility of using them with existing `unittest.TestCase` or TestSlide's own test runner let users get its benefits without requiring refactoring existing code. + +## Quickstart + +Install: + +``` +pip install TestSlide +``` + +Scaffold the code you want to test `backup.py`: + +```python +class Backup: + def delete(self, path): + pass +``` + +Write a test case `backup_test.py` describing the expected behavior: + +```python +import testslide, backup, storage + +class TestBackupDelete(testslide.TestCase): + def setUp(self): + super().setUp() + self.storage_mock = testslide.StrictMock(storage.Client) + # Makes storage.Client(timeout=60) return self.storage_mock + self.mock_constructor(storage, 'Client')\ + .for_call(timeout=60)\ + .to_return_value(self.storage_mock) + + def test_delete_from_storage(self): + # Set behavior and assertion for the call at the mock + self.mock_callable(self.storage_mock, 'delete')\ + .for_call('/file/to/delete')\ + .to_return_value(True)\ + .and_assert_called_once() + backup.Backup().delete('/file/to/delete') +``` + +TestSlide's `StrictMock`, `mock_constructor()` and `mock_callable()` are seamlessly integrated with Python's TestCase. + +Run the test and see the failure: + +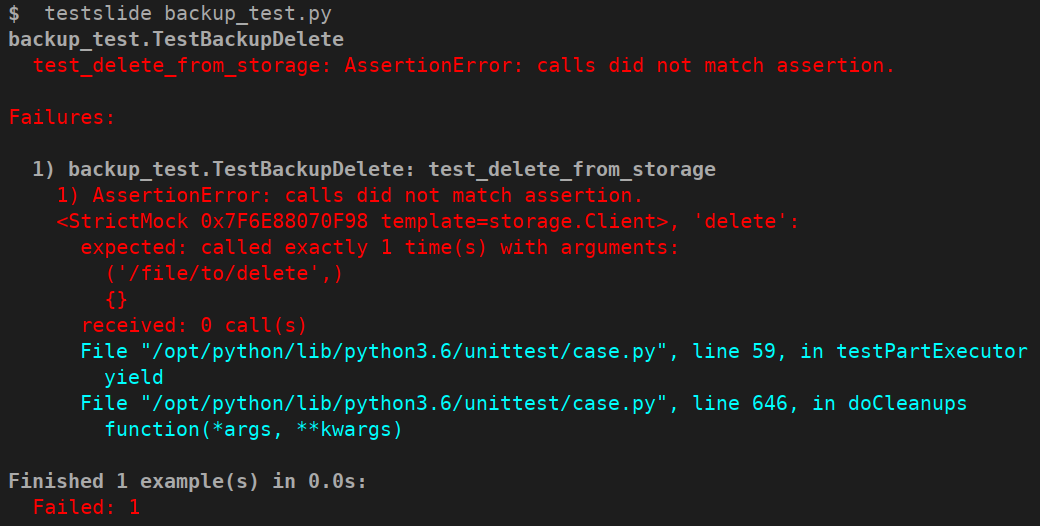 + +TestSlide's mocks failure messages guide you towards the solution, that you can now implement: + +```python +import storage + +class Backup: + def __init__(self): + self.storage = storage.Client(timeout=60) + + def delete(self, path): + self.storage.delete(path) +``` + +And watch the test go green: + +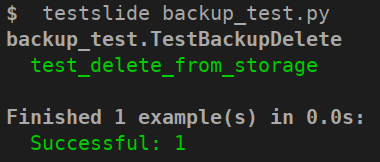 + +It is all about letting the failure messages guide you towards the solution. There's a plethora of validation inside TestSlide's mocks, so you can trust they will help you iterate quickly when writing code and also cover you when breaking changes are introduced. + +## Full documentation + +There's a lot more that TestSlide can offer, please check the full documentation at https://testslide.readthedocs.io/ to learn more. + +## Requirements + +* Linux +* Python 3 + +## Join the TestSlide community + +TestSlide is open source software, contributions are very welcome! + +See the [CONTRIBUTING](CONTRIBUTING.md) file for how to help out. + +## License + +TestSlide is MIT licensed, as found in the [LICENSE](LICENSE) file. + + +## Terms of Use + +https://opensource.facebook.com/legal/terms + + +## Privacy Policy + +https://opensource.facebook.com/legal/privacy + +%prep +%autosetup -n TestSlide-2.7.0 + +%build +%py3_build + +%install +%py3_install +install -d -m755 %{buildroot}/%{_pkgdocdir} +if [ -d doc ]; then cp -arf doc %{buildroot}/%{_pkgdocdir}; fi +if [ -d docs ]; then cp -arf docs %{buildroot}/%{_pkgdocdir}; fi +if [ -d example ]; then cp -arf example %{buildroot}/%{_pkgdocdir}; fi +if [ -d examples ]; then cp -arf examples %{buildroot}/%{_pkgdocdir}; fi +pushd %{buildroot} +if [ -d usr/lib ]; then + find usr/lib -type f -printf "/%h/%f\n" >> filelist.lst +fi +if [ -d usr/lib64 ]; then + find usr/lib64 -type f -printf "/%h/%f\n" >> filelist.lst +fi +if [ -d usr/bin ]; then + find usr/bin -type f -printf "/%h/%f\n" >> filelist.lst +fi +if [ -d usr/sbin ]; then + find usr/sbin -type f -printf "/%h/%f\n" >> filelist.lst +fi +touch doclist.lst +if [ -d usr/share/man ]; then + find usr/share/man -type f -printf "/%h/%f.gz\n" >> doclist.lst +fi +popd +mv %{buildroot}/filelist.lst . +mv %{buildroot}/doclist.lst . + +%files -n python3-TestSlide -f filelist.lst +%dir %{python3_sitelib}/* + +%files help -f doclist.lst +%{_docdir}/* + +%changelog +* Mon Mar 06 2023 Python_Bot <Python_Bot@openeuler.org> - 2.7.0-1 +- Package Spec generated @@ -0,0 +1 @@ +2f9935845631bd01299955e0cbffa33c TestSlide-2.7.0.tar.gz |