diff options
-rw-r--r-- | .gitignore | 1 | ||||
-rw-r--r-- | python-django-translations.spec | 699 | ||||
-rw-r--r-- | sources | 1 |
3 files changed, 701 insertions, 0 deletions
@@ -0,0 +1 @@ +/django-translations-1.3.0.tar.gz diff --git a/python-django-translations.spec b/python-django-translations.spec new file mode 100644 index 0000000..de7f420 --- /dev/null +++ b/python-django-translations.spec @@ -0,0 +1,699 @@ +%global _empty_manifest_terminate_build 0 +Name: python-django-translations +Version: 1.3.0 +Release: 1 +Summary: Django model translation for perfectionists with deadlines. +License: BSD License +URL: https://github.com/bbmokhtari/django-translations +Source0: https://mirrors.nju.edu.cn/pypi/web/packages/ed/6c/ff685532793240ede017a45aa5eeafcc1ffc7eb30c5c587fead6bc138158/django-translations-1.3.0.tar.gz +BuildArch: noarch + + +%description +# Django Translations + +[](https://travis-ci.com/bbmokhtari/django-translations) +[](https://pypi.org/project/django-translations/) +[](https://pypi.org/project/django-translations/) + +Django model translation for perfectionists with deadlines. + +## Goal + +There are two types of content, each of which has its own challenges for translation: + +- Static content: This is the content which is defined in the code. + _e.g. "Please enter a valid email address."_ + + Django already provides a + [solution](https://docs.djangoproject.com/en/2.2/topics/i18n/translation/) + for translating static content. + +- Dynamic content: This is the content which is stored in the database. + _(We can't know it beforehand!)_ + + Django Translations provides a solution + for translating dynamic content. + +## Compatibility + +Currently, this project is incompatible with PostgreSQL. + +## Requirements + +- Python (\>=3.6, \<4) +- Django (\>=2.2, \<4) + +## Installation + +1. Install Django Translations using pip: + + ``` bash + $ pip install django-translations + ``` + +2. Add `translations` to the `INSTALLED_APPS` in the settings of your + project: + + ``` python + INSTALLED_APPS += [ + 'translations', + ] + ``` + +3. Run `migrate`: + + ``` bash + $ python manage.py migrate + ``` + +4. Configure Django internationalization and localization settings: + + ``` python + USE_I18N = True # use internationalization + USE_L10N = True # use localization + + MIDDLEWARE += [ # locale middleware + 'django.middleware.locale.LocaleMiddleware', + ] + + LANGUAGE_CODE = 'en-us' # default (fallback) language + LANGUAGES = ( # supported languages + ('en', 'English'), + ('en-gb', 'English (Great Britain)'), + ('de', 'German'), + ('tr', 'Turkish'), + ) + ``` + + Please note that these settings are for Django itself. + +## Basic Usage + +### Model + +Inherit `Translatable` in any model you want translated: + +``` python +from translations.models import Translatable + +class Continent(Translatable): + code = models.Charfield(...) + name = models.Charfield(...) + denonym = models.Charfield(...) + + class TranslatableMeta: + fields = ['name', 'denonym'] +``` + +No migrations needed afterwards. + +### Admin + +Use the admin extensions: + +``` python +from translations.admin import TranslatableAdmin, TranslationInline + +class ContinentAdmin(TranslatableAdmin): + inlines = [TranslationInline,] +``` + +This provides specialized translation inlines for the model. + +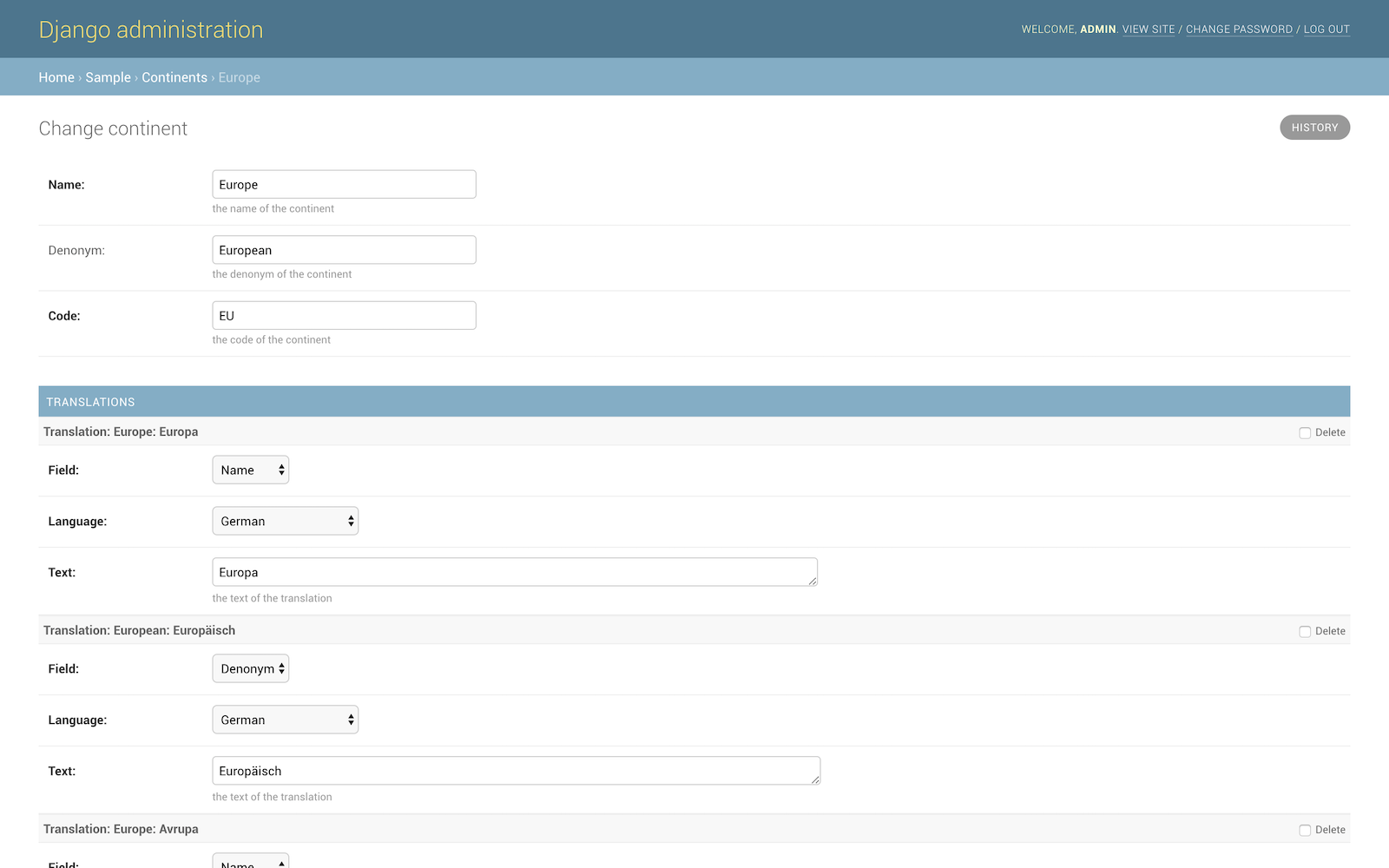 + +## QuerySet + +Use the queryset extensions: + +``` python +>>> from sample.models import Continent +>>> continents = Continent.objects.all( +... ).distinct( # familiar distinct +... ).probe(['en', 'de'] # probe (filter, exclude, etc.) in English and German +... ).filter( # familiar filtering +... countries__cities__name__startswith='Köln' +... ).translate('de' # translate the results in German +... ).translate_related( # translate these relations as well +... 'countries', 'countries__cities', +... ) +>>> print(continents) +<TranslatableQuerySet [ + <Continent: Europa>, +]> +>>> print(continents[0].countries.all()) +<TranslatableQuerySet [ + <Country: Deutschland>, +]> +>>> print(continents[0].countries.all()[0].cities.all()) +<TranslatableQuerySet [ + <City: Köln>, +]> +``` + +This provides a powerful yet familiar interface to work with the querysets. + +## Context + +Use the translation context: + +``` python +>>> from translations.context import Context +>>> from sample.models import Continent +>>> continents = Continent.objects.all() +>>> relations = ('countries', 'countries__cities',) +>>> with Context(continents, *relations) as context: +... context.read('de') # read the translations onto the context +... print(':') # use the objects like before +... print(continents) +... print(continents[0].countries.all()) +... print(continents[0].countries.all()[0].cities.all()) +... +... continents[0].countries.all()[0].name = 'Change the name' +... context.update('de') # update the translations from the context +... +... context.delete('de') # delete the translations of the context +... +... context.reset() # reset the translations of the context +... print(':') # use the objects like before +... print(continents) +... print(continents[0].countries.all()) +... print(continents[0].countries.all()[0].cities.all()) +: +<TranslatableQuerySet [ + <Continent: Asien>, + <Continent: Europa>, +]> +<TranslatableQuerySet [ + <Country: Deutschland>, +]> +<TranslatableQuerySet [ + <City: Köln>, +]> +: +<TranslatableQuerySet [ + <Continent: Asia>, + <Continent: Europe>, +]> +<TranslatableQuerySet [ + <Country: Germany>, +]> +<TranslatableQuerySet [ + <City: Cologne>, +]> +``` + +This can CRUD the translations of any objects (instance, queryset, list) and their relations. + +## Documentation + +For more interesting capabilities browse through the +[documentation](http://bbmokhtari.github.io/django-translations). + +## Support the project + +To support the project you can: +- ⭐️: [Star](http://github.com/bbmokhtari/django-translations/) it on GitHub. +- 💻: [Contribute](https://bbmokhtari.github.io/django-translations/contribution.html) to the code base. +- ☕️: [Buy](https://bbmokhtari.github.io/django-translations/donation.html) the maintainers coffee. + + + + +%package -n python3-django-translations +Summary: Django model translation for perfectionists with deadlines. +Provides: python-django-translations +BuildRequires: python3-devel +BuildRequires: python3-setuptools +BuildRequires: python3-pip +%description -n python3-django-translations +# Django Translations + +[](https://travis-ci.com/bbmokhtari/django-translations) +[](https://pypi.org/project/django-translations/) +[](https://pypi.org/project/django-translations/) + +Django model translation for perfectionists with deadlines. + +## Goal + +There are two types of content, each of which has its own challenges for translation: + +- Static content: This is the content which is defined in the code. + _e.g. "Please enter a valid email address."_ + + Django already provides a + [solution](https://docs.djangoproject.com/en/2.2/topics/i18n/translation/) + for translating static content. + +- Dynamic content: This is the content which is stored in the database. + _(We can't know it beforehand!)_ + + Django Translations provides a solution + for translating dynamic content. + +## Compatibility + +Currently, this project is incompatible with PostgreSQL. + +## Requirements + +- Python (\>=3.6, \<4) +- Django (\>=2.2, \<4) + +## Installation + +1. Install Django Translations using pip: + + ``` bash + $ pip install django-translations + ``` + +2. Add `translations` to the `INSTALLED_APPS` in the settings of your + project: + + ``` python + INSTALLED_APPS += [ + 'translations', + ] + ``` + +3. Run `migrate`: + + ``` bash + $ python manage.py migrate + ``` + +4. Configure Django internationalization and localization settings: + + ``` python + USE_I18N = True # use internationalization + USE_L10N = True # use localization + + MIDDLEWARE += [ # locale middleware + 'django.middleware.locale.LocaleMiddleware', + ] + + LANGUAGE_CODE = 'en-us' # default (fallback) language + LANGUAGES = ( # supported languages + ('en', 'English'), + ('en-gb', 'English (Great Britain)'), + ('de', 'German'), + ('tr', 'Turkish'), + ) + ``` + + Please note that these settings are for Django itself. + +## Basic Usage + +### Model + +Inherit `Translatable` in any model you want translated: + +``` python +from translations.models import Translatable + +class Continent(Translatable): + code = models.Charfield(...) + name = models.Charfield(...) + denonym = models.Charfield(...) + + class TranslatableMeta: + fields = ['name', 'denonym'] +``` + +No migrations needed afterwards. + +### Admin + +Use the admin extensions: + +``` python +from translations.admin import TranslatableAdmin, TranslationInline + +class ContinentAdmin(TranslatableAdmin): + inlines = [TranslationInline,] +``` + +This provides specialized translation inlines for the model. + +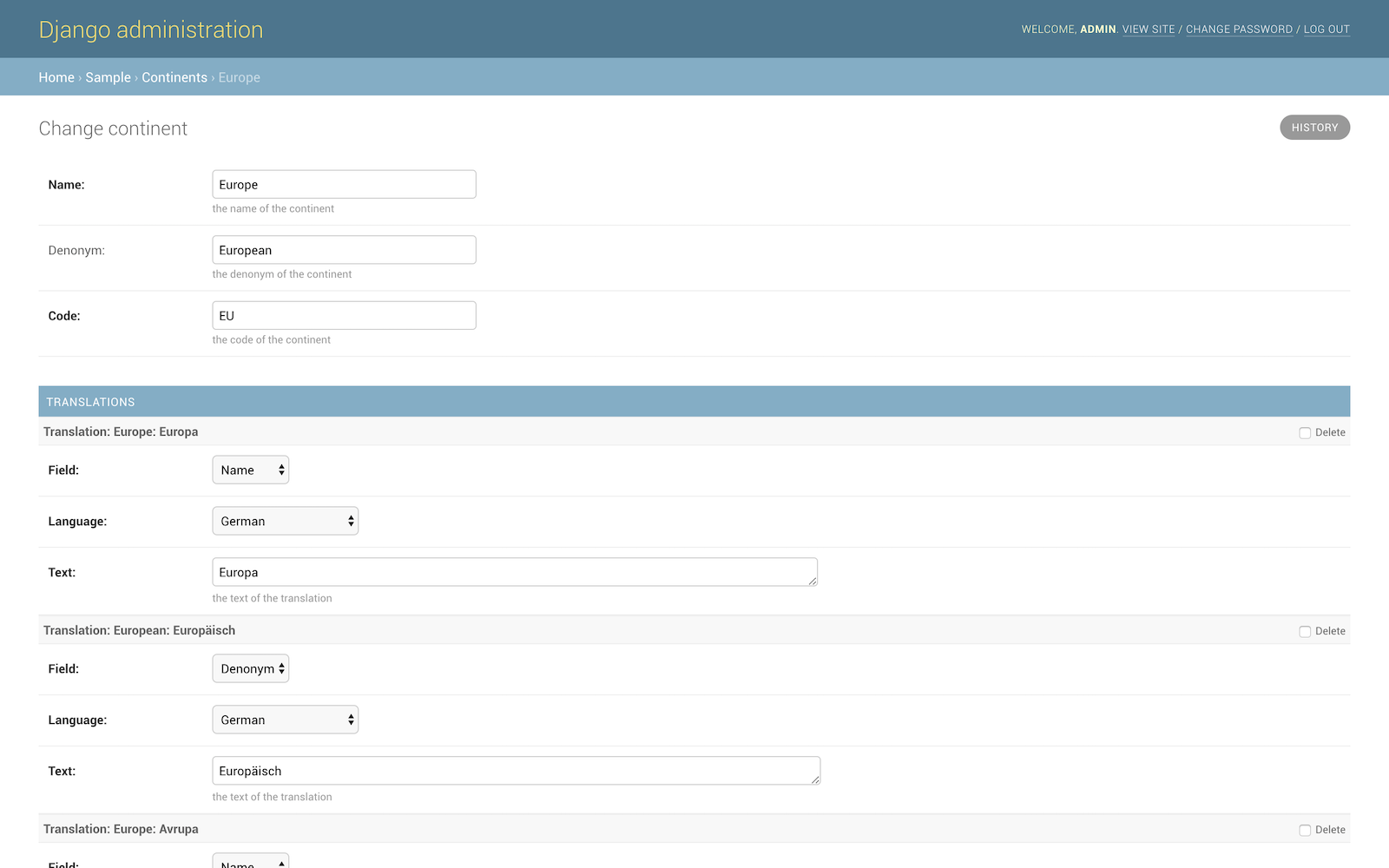 + +## QuerySet + +Use the queryset extensions: + +``` python +>>> from sample.models import Continent +>>> continents = Continent.objects.all( +... ).distinct( # familiar distinct +... ).probe(['en', 'de'] # probe (filter, exclude, etc.) in English and German +... ).filter( # familiar filtering +... countries__cities__name__startswith='Köln' +... ).translate('de' # translate the results in German +... ).translate_related( # translate these relations as well +... 'countries', 'countries__cities', +... ) +>>> print(continents) +<TranslatableQuerySet [ + <Continent: Europa>, +]> +>>> print(continents[0].countries.all()) +<TranslatableQuerySet [ + <Country: Deutschland>, +]> +>>> print(continents[0].countries.all()[0].cities.all()) +<TranslatableQuerySet [ + <City: Köln>, +]> +``` + +This provides a powerful yet familiar interface to work with the querysets. + +## Context + +Use the translation context: + +``` python +>>> from translations.context import Context +>>> from sample.models import Continent +>>> continents = Continent.objects.all() +>>> relations = ('countries', 'countries__cities',) +>>> with Context(continents, *relations) as context: +... context.read('de') # read the translations onto the context +... print(':') # use the objects like before +... print(continents) +... print(continents[0].countries.all()) +... print(continents[0].countries.all()[0].cities.all()) +... +... continents[0].countries.all()[0].name = 'Change the name' +... context.update('de') # update the translations from the context +... +... context.delete('de') # delete the translations of the context +... +... context.reset() # reset the translations of the context +... print(':') # use the objects like before +... print(continents) +... print(continents[0].countries.all()) +... print(continents[0].countries.all()[0].cities.all()) +: +<TranslatableQuerySet [ + <Continent: Asien>, + <Continent: Europa>, +]> +<TranslatableQuerySet [ + <Country: Deutschland>, +]> +<TranslatableQuerySet [ + <City: Köln>, +]> +: +<TranslatableQuerySet [ + <Continent: Asia>, + <Continent: Europe>, +]> +<TranslatableQuerySet [ + <Country: Germany>, +]> +<TranslatableQuerySet [ + <City: Cologne>, +]> +``` + +This can CRUD the translations of any objects (instance, queryset, list) and their relations. + +## Documentation + +For more interesting capabilities browse through the +[documentation](http://bbmokhtari.github.io/django-translations). + +## Support the project + +To support the project you can: +- ⭐️: [Star](http://github.com/bbmokhtari/django-translations/) it on GitHub. +- 💻: [Contribute](https://bbmokhtari.github.io/django-translations/contribution.html) to the code base. +- ☕️: [Buy](https://bbmokhtari.github.io/django-translations/donation.html) the maintainers coffee. + + + + +%package help +Summary: Development documents and examples for django-translations +Provides: python3-django-translations-doc +%description help +# Django Translations + +[](https://travis-ci.com/bbmokhtari/django-translations) +[](https://pypi.org/project/django-translations/) +[](https://pypi.org/project/django-translations/) + +Django model translation for perfectionists with deadlines. + +## Goal + +There are two types of content, each of which has its own challenges for translation: + +- Static content: This is the content which is defined in the code. + _e.g. "Please enter a valid email address."_ + + Django already provides a + [solution](https://docs.djangoproject.com/en/2.2/topics/i18n/translation/) + for translating static content. + +- Dynamic content: This is the content which is stored in the database. + _(We can't know it beforehand!)_ + + Django Translations provides a solution + for translating dynamic content. + +## Compatibility + +Currently, this project is incompatible with PostgreSQL. + +## Requirements + +- Python (\>=3.6, \<4) +- Django (\>=2.2, \<4) + +## Installation + +1. Install Django Translations using pip: + + ``` bash + $ pip install django-translations + ``` + +2. Add `translations` to the `INSTALLED_APPS` in the settings of your + project: + + ``` python + INSTALLED_APPS += [ + 'translations', + ] + ``` + +3. Run `migrate`: + + ``` bash + $ python manage.py migrate + ``` + +4. Configure Django internationalization and localization settings: + + ``` python + USE_I18N = True # use internationalization + USE_L10N = True # use localization + + MIDDLEWARE += [ # locale middleware + 'django.middleware.locale.LocaleMiddleware', + ] + + LANGUAGE_CODE = 'en-us' # default (fallback) language + LANGUAGES = ( # supported languages + ('en', 'English'), + ('en-gb', 'English (Great Britain)'), + ('de', 'German'), + ('tr', 'Turkish'), + ) + ``` + + Please note that these settings are for Django itself. + +## Basic Usage + +### Model + +Inherit `Translatable` in any model you want translated: + +``` python +from translations.models import Translatable + +class Continent(Translatable): + code = models.Charfield(...) + name = models.Charfield(...) + denonym = models.Charfield(...) + + class TranslatableMeta: + fields = ['name', 'denonym'] +``` + +No migrations needed afterwards. + +### Admin + +Use the admin extensions: + +``` python +from translations.admin import TranslatableAdmin, TranslationInline + +class ContinentAdmin(TranslatableAdmin): + inlines = [TranslationInline,] +``` + +This provides specialized translation inlines for the model. + +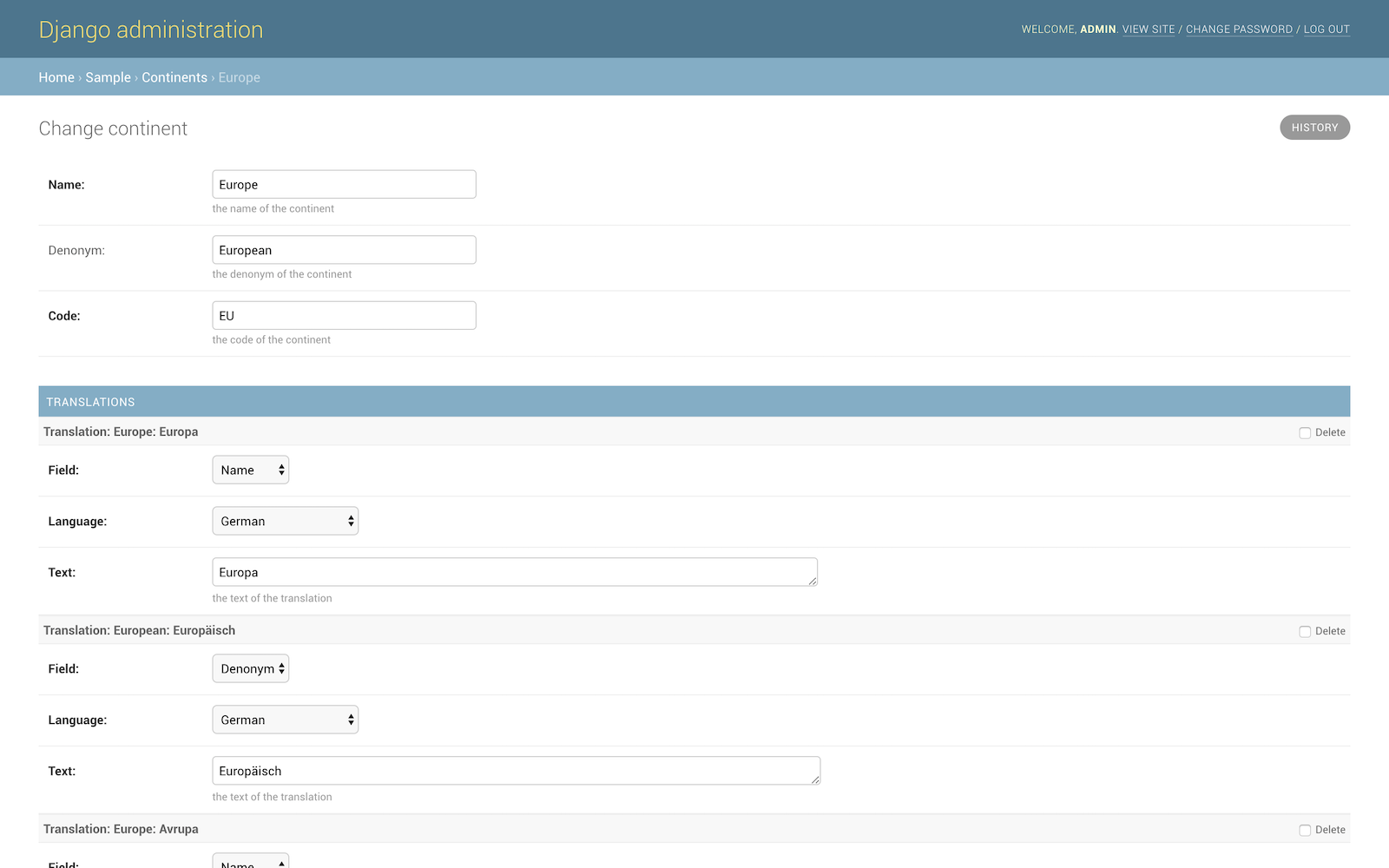 + +## QuerySet + +Use the queryset extensions: + +``` python +>>> from sample.models import Continent +>>> continents = Continent.objects.all( +... ).distinct( # familiar distinct +... ).probe(['en', 'de'] # probe (filter, exclude, etc.) in English and German +... ).filter( # familiar filtering +... countries__cities__name__startswith='Köln' +... ).translate('de' # translate the results in German +... ).translate_related( # translate these relations as well +... 'countries', 'countries__cities', +... ) +>>> print(continents) +<TranslatableQuerySet [ + <Continent: Europa>, +]> +>>> print(continents[0].countries.all()) +<TranslatableQuerySet [ + <Country: Deutschland>, +]> +>>> print(continents[0].countries.all()[0].cities.all()) +<TranslatableQuerySet [ + <City: Köln>, +]> +``` + +This provides a powerful yet familiar interface to work with the querysets. + +## Context + +Use the translation context: + +``` python +>>> from translations.context import Context +>>> from sample.models import Continent +>>> continents = Continent.objects.all() +>>> relations = ('countries', 'countries__cities',) +>>> with Context(continents, *relations) as context: +... context.read('de') # read the translations onto the context +... print(':') # use the objects like before +... print(continents) +... print(continents[0].countries.all()) +... print(continents[0].countries.all()[0].cities.all()) +... +... continents[0].countries.all()[0].name = 'Change the name' +... context.update('de') # update the translations from the context +... +... context.delete('de') # delete the translations of the context +... +... context.reset() # reset the translations of the context +... print(':') # use the objects like before +... print(continents) +... print(continents[0].countries.all()) +... print(continents[0].countries.all()[0].cities.all()) +: +<TranslatableQuerySet [ + <Continent: Asien>, + <Continent: Europa>, +]> +<TranslatableQuerySet [ + <Country: Deutschland>, +]> +<TranslatableQuerySet [ + <City: Köln>, +]> +: +<TranslatableQuerySet [ + <Continent: Asia>, + <Continent: Europe>, +]> +<TranslatableQuerySet [ + <Country: Germany>, +]> +<TranslatableQuerySet [ + <City: Cologne>, +]> +``` + +This can CRUD the translations of any objects (instance, queryset, list) and their relations. + +## Documentation + +For more interesting capabilities browse through the +[documentation](http://bbmokhtari.github.io/django-translations). + +## Support the project + +To support the project you can: +- ⭐️: [Star](http://github.com/bbmokhtari/django-translations/) it on GitHub. +- 💻: [Contribute](https://bbmokhtari.github.io/django-translations/contribution.html) to the code base. +- ☕️: [Buy](https://bbmokhtari.github.io/django-translations/donation.html) the maintainers coffee. + + + + +%prep +%autosetup -n django-translations-1.3.0 + +%build +%py3_build + +%install +%py3_install +install -d -m755 %{buildroot}/%{_pkgdocdir} +if [ -d doc ]; then cp -arf doc %{buildroot}/%{_pkgdocdir}; fi +if [ -d docs ]; then cp -arf docs %{buildroot}/%{_pkgdocdir}; fi +if [ -d example ]; then cp -arf example %{buildroot}/%{_pkgdocdir}; fi +if [ -d examples ]; then cp -arf examples %{buildroot}/%{_pkgdocdir}; fi +pushd %{buildroot} +if [ -d usr/lib ]; then + find usr/lib -type f -printf "/%h/%f\n" >> filelist.lst +fi +if [ -d usr/lib64 ]; then + find usr/lib64 -type f -printf "/%h/%f\n" >> filelist.lst +fi +if [ -d usr/bin ]; then + find usr/bin -type f -printf "/%h/%f\n" >> filelist.lst +fi +if [ -d usr/sbin ]; then + find usr/sbin -type f -printf "/%h/%f\n" >> filelist.lst +fi +touch doclist.lst +if [ -d usr/share/man ]; then + find usr/share/man -type f -printf "/%h/%f.gz\n" >> doclist.lst +fi +popd +mv %{buildroot}/filelist.lst . +mv %{buildroot}/doclist.lst . + +%files -n python3-django-translations -f filelist.lst +%dir %{python3_sitelib}/* + +%files help -f doclist.lst +%{_docdir}/* + +%changelog +* Mon May 15 2023 Python_Bot <Python_Bot@openeuler.org> - 1.3.0-1 +- Package Spec generated @@ -0,0 +1 @@ +ebad0251a1b860fbb4b7dca5e2a85590 django-translations-1.3.0.tar.gz |