%global _empty_manifest_terminate_build 0
Name: python-ethereum-kms-signer
Version: 0.1.6
Release: 1
Summary: Sign ETH transactions with keys stored in AWS KMS.
License: MIT
URL: https://github.com/meetmangukiya/ethereum_kms_signer
Source0: https://mirrors.aliyun.com/pypi/web/packages/ee/39/1c472f6d204aeae65e5b949997030aab3ad30f876abc4242577d28de2977/ethereum_kms_signer-0.1.6.tar.gz
BuildArch: noarch
Requires: python3-boto3
Requires: python3-boto3-stubs[kms]
Requires: python3-eth-account
Requires: python3-eth-utils
Requires: python3-fire
Requires: python3-hexbytes
Requires: python3-pyasn1
Requires: python3-pycryptodome
Requires: python3-toolz
%description
# Ethereum KMS Signer
Sign ETH transactions with keys stored in AWS KMS
* Free software: MIT
* Documentation:
## Features
* Sign Transactions
## Video Demo
[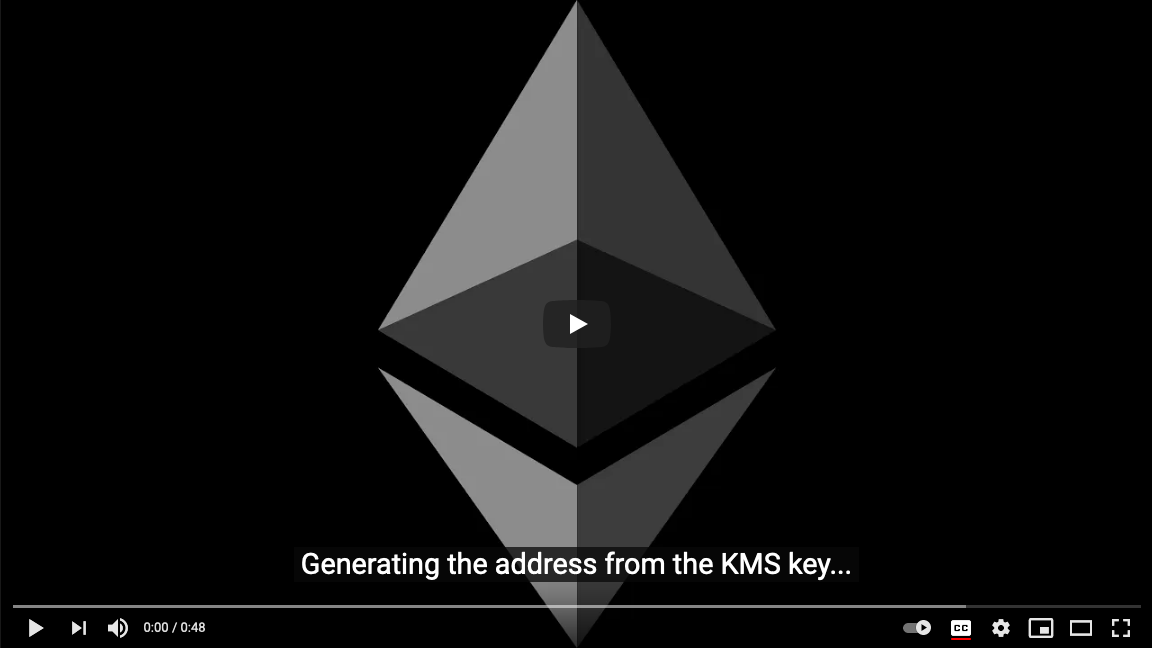](https://youtu.be/fZ-mtMb2BjY "Python Ethereum KMS Signer Demo")
## Why?
In the crypto world, all the assets, tokens, crypto you might own is
protected by the secrecy of the private key. This leads to a single point
of failure in cases of leaking of private keys or losing keys because of
lack of backup or any number of reasons. It becomes even harder when you want
to share these keys as an organization among many individuals.
Using something like AWS KMS can help with that and can provide full benefits
of all the security features it provides. Sigantures can be created without the key
ever leaving the AWS's infrastructure and could be effectively shared among individuals.
This library provides a simple and an easy-to-use API for using AWS KMS to sign ethereum
transactions and an easy integration with `web3.py` making it practical for using KMS to
manage your private keys.
## Quickstart
### Get ethereum address from KMS key
```python
from ethereum_kms_signer import get_eth_address
address = get_eth_address('THE-AWS-KMS-ID')
print(address)
```
### Sign a transaction object with KMS key
```python
from ethereum_kms_signer import sign_transaction
dai_txn = dai.functions.transfer(
web3.toChecksumAddress(to_address.lower()), amount
).buildTransaction(
{
"nonce": nonce,
}
)
# Signing the transaction with KMS key
signed_tx = sign_transaction(dai_txn, key_id)
# send transaction
tx_hash = web3.eth.sendRawTransaction(signed_tx.rawTransaction)
```
### Provisioning AWS KMS key with terraform
An `ECC_SECG_P256K1` key can be provisioned using terraform by using the following
configuration along with the aws provider. More details can be found on
[provider docs]()
```tf
resource "aws_kms_key" "my_very_secret_eth_account" {
description = "ETH account #1"
key_usage = "SIGN_VERIFY"
customer_master_key_spec = "ECC_SECG_P256K1"
}
resource "aws_kms_alias" "my_very_secret_eth_account" {
name = "eth-account-1"
target_key_id = aws_kms_key.my_very_secret_eth_account.id
}
```
## Examples
Few examples can be found [here](https://github.com/meetmangukiya/ethereum-kms-signer/tree/main/examples).
## Credits
This package was created with [Cookiecutter](https://github.com/audreyr/cookiecutter) and the [zillionare/cookiecutter-pypackage](https://github.com/zillionare/cookiecutter-pypackage) project template.
[This article](https://luhenning.medium.com/the-dark-side-of-the-elliptic-curve-signing-ethereum-transactions-with-aws-kms-in-javascript-83610d9a6f81) has served as a good resource for implementing the functionality
%package -n python3-ethereum-kms-signer
Summary: Sign ETH transactions with keys stored in AWS KMS.
Provides: python-ethereum-kms-signer
BuildRequires: python3-devel
BuildRequires: python3-setuptools
BuildRequires: python3-pip
%description -n python3-ethereum-kms-signer
# Ethereum KMS Signer
Sign ETH transactions with keys stored in AWS KMS
* Free software: MIT
* Documentation:
## Features
* Sign Transactions
## Video Demo
[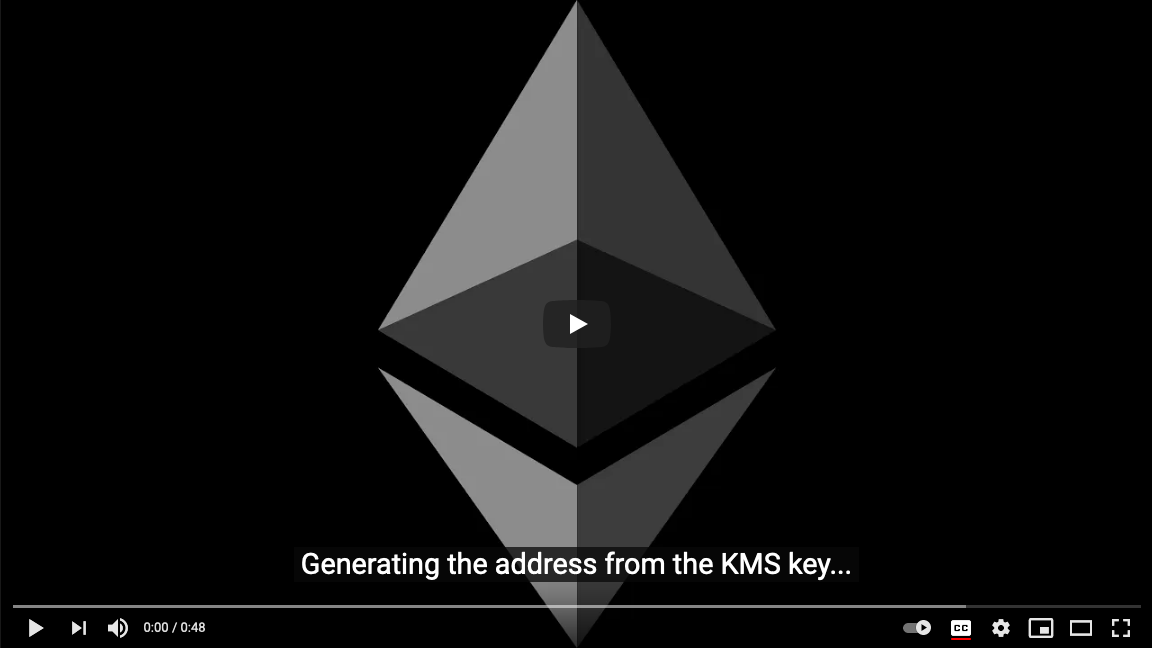](https://youtu.be/fZ-mtMb2BjY "Python Ethereum KMS Signer Demo")
## Why?
In the crypto world, all the assets, tokens, crypto you might own is
protected by the secrecy of the private key. This leads to a single point
of failure in cases of leaking of private keys or losing keys because of
lack of backup or any number of reasons. It becomes even harder when you want
to share these keys as an organization among many individuals.
Using something like AWS KMS can help with that and can provide full benefits
of all the security features it provides. Sigantures can be created without the key
ever leaving the AWS's infrastructure and could be effectively shared among individuals.
This library provides a simple and an easy-to-use API for using AWS KMS to sign ethereum
transactions and an easy integration with `web3.py` making it practical for using KMS to
manage your private keys.
## Quickstart
### Get ethereum address from KMS key
```python
from ethereum_kms_signer import get_eth_address
address = get_eth_address('THE-AWS-KMS-ID')
print(address)
```
### Sign a transaction object with KMS key
```python
from ethereum_kms_signer import sign_transaction
dai_txn = dai.functions.transfer(
web3.toChecksumAddress(to_address.lower()), amount
).buildTransaction(
{
"nonce": nonce,
}
)
# Signing the transaction with KMS key
signed_tx = sign_transaction(dai_txn, key_id)
# send transaction
tx_hash = web3.eth.sendRawTransaction(signed_tx.rawTransaction)
```
### Provisioning AWS KMS key with terraform
An `ECC_SECG_P256K1` key can be provisioned using terraform by using the following
configuration along with the aws provider. More details can be found on
[provider docs]()
```tf
resource "aws_kms_key" "my_very_secret_eth_account" {
description = "ETH account #1"
key_usage = "SIGN_VERIFY"
customer_master_key_spec = "ECC_SECG_P256K1"
}
resource "aws_kms_alias" "my_very_secret_eth_account" {
name = "eth-account-1"
target_key_id = aws_kms_key.my_very_secret_eth_account.id
}
```
## Examples
Few examples can be found [here](https://github.com/meetmangukiya/ethereum-kms-signer/tree/main/examples).
## Credits
This package was created with [Cookiecutter](https://github.com/audreyr/cookiecutter) and the [zillionare/cookiecutter-pypackage](https://github.com/zillionare/cookiecutter-pypackage) project template.
[This article](https://luhenning.medium.com/the-dark-side-of-the-elliptic-curve-signing-ethereum-transactions-with-aws-kms-in-javascript-83610d9a6f81) has served as a good resource for implementing the functionality
%package help
Summary: Development documents and examples for ethereum-kms-signer
Provides: python3-ethereum-kms-signer-doc
%description help
# Ethereum KMS Signer
Sign ETH transactions with keys stored in AWS KMS
* Free software: MIT
* Documentation:
## Features
* Sign Transactions
## Video Demo
[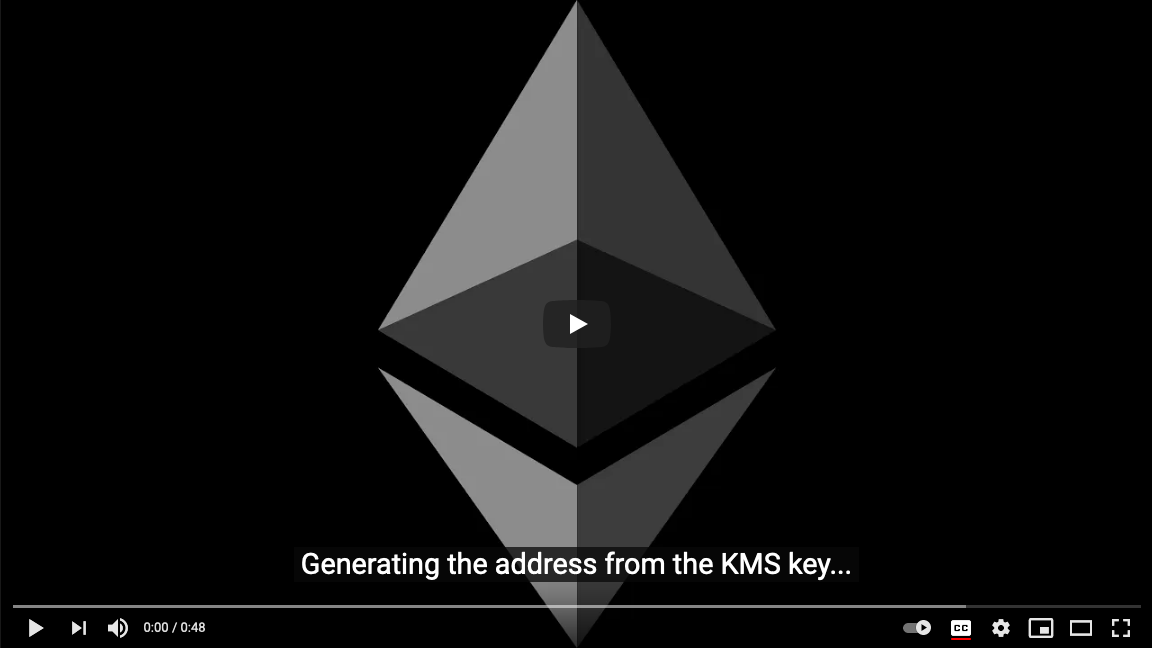](https://youtu.be/fZ-mtMb2BjY "Python Ethereum KMS Signer Demo")
## Why?
In the crypto world, all the assets, tokens, crypto you might own is
protected by the secrecy of the private key. This leads to a single point
of failure in cases of leaking of private keys or losing keys because of
lack of backup or any number of reasons. It becomes even harder when you want
to share these keys as an organization among many individuals.
Using something like AWS KMS can help with that and can provide full benefits
of all the security features it provides. Sigantures can be created without the key
ever leaving the AWS's infrastructure and could be effectively shared among individuals.
This library provides a simple and an easy-to-use API for using AWS KMS to sign ethereum
transactions and an easy integration with `web3.py` making it practical for using KMS to
manage your private keys.
## Quickstart
### Get ethereum address from KMS key
```python
from ethereum_kms_signer import get_eth_address
address = get_eth_address('THE-AWS-KMS-ID')
print(address)
```
### Sign a transaction object with KMS key
```python
from ethereum_kms_signer import sign_transaction
dai_txn = dai.functions.transfer(
web3.toChecksumAddress(to_address.lower()), amount
).buildTransaction(
{
"nonce": nonce,
}
)
# Signing the transaction with KMS key
signed_tx = sign_transaction(dai_txn, key_id)
# send transaction
tx_hash = web3.eth.sendRawTransaction(signed_tx.rawTransaction)
```
### Provisioning AWS KMS key with terraform
An `ECC_SECG_P256K1` key can be provisioned using terraform by using the following
configuration along with the aws provider. More details can be found on
[provider docs]()
```tf
resource "aws_kms_key" "my_very_secret_eth_account" {
description = "ETH account #1"
key_usage = "SIGN_VERIFY"
customer_master_key_spec = "ECC_SECG_P256K1"
}
resource "aws_kms_alias" "my_very_secret_eth_account" {
name = "eth-account-1"
target_key_id = aws_kms_key.my_very_secret_eth_account.id
}
```
## Examples
Few examples can be found [here](https://github.com/meetmangukiya/ethereum-kms-signer/tree/main/examples).
## Credits
This package was created with [Cookiecutter](https://github.com/audreyr/cookiecutter) and the [zillionare/cookiecutter-pypackage](https://github.com/zillionare/cookiecutter-pypackage) project template.
[This article](https://luhenning.medium.com/the-dark-side-of-the-elliptic-curve-signing-ethereum-transactions-with-aws-kms-in-javascript-83610d9a6f81) has served as a good resource for implementing the functionality
%prep
%autosetup -n ethereum_kms_signer-0.1.6
%build
%py3_build
%install
%py3_install
install -d -m755 %{buildroot}/%{_pkgdocdir}
if [ -d doc ]; then cp -arf doc %{buildroot}/%{_pkgdocdir}; fi
if [ -d docs ]; then cp -arf docs %{buildroot}/%{_pkgdocdir}; fi
if [ -d example ]; then cp -arf example %{buildroot}/%{_pkgdocdir}; fi
if [ -d examples ]; then cp -arf examples %{buildroot}/%{_pkgdocdir}; fi
pushd %{buildroot}
if [ -d usr/lib ]; then
find usr/lib -type f -printf "\"/%h/%f\"\n" >> filelist.lst
fi
if [ -d usr/lib64 ]; then
find usr/lib64 -type f -printf "\"/%h/%f\"\n" >> filelist.lst
fi
if [ -d usr/bin ]; then
find usr/bin -type f -printf "\"/%h/%f\"\n" >> filelist.lst
fi
if [ -d usr/sbin ]; then
find usr/sbin -type f -printf "\"/%h/%f\"\n" >> filelist.lst
fi
touch doclist.lst
if [ -d usr/share/man ]; then
find usr/share/man -type f -printf "\"/%h/%f.gz\"\n" >> doclist.lst
fi
popd
mv %{buildroot}/filelist.lst .
mv %{buildroot}/doclist.lst .
%files -n python3-ethereum-kms-signer -f filelist.lst
%dir %{python3_sitelib}/*
%files help -f doclist.lst
%{_docdir}/*
%changelog
* Tue Jun 20 2023 Python_Bot - 0.1.6-1
- Package Spec generated