%global _empty_manifest_terminate_build 0
Name: python-alive-progress
Version: 3.1.1
Release: 1
Summary: A new kind of Progress Bar, with real-time throughput, ETA, and very cool animations!
License: MIT
URL: https://github.com/rsalmei/alive-progress
Source0: https://mirrors.nju.edu.cn/pypi/web/packages/db/ad/f67b278cc654d51872dc1ed437f383522f9b715043ff80a3401fcdebf98e/alive-progress-3.1.1.tar.gz
BuildArch: noarch
Requires: python3-about-time
Requires: python3-grapheme
%description
## Using `alive-progress`
### Get it
Just install with pip:
```sh
❯ pip install alive-progress
```
### Try it
If you're wondering what styles are builtin, it's `showtime`! ;)
```python
from alive_progress.styles import showtime
showtime()
```
> Note: Please disregard the path in the animated gif below, the correct one is above. These long gifs are very time-consuming to generate, so I can't make another on every single change. Thanks for your understanding.
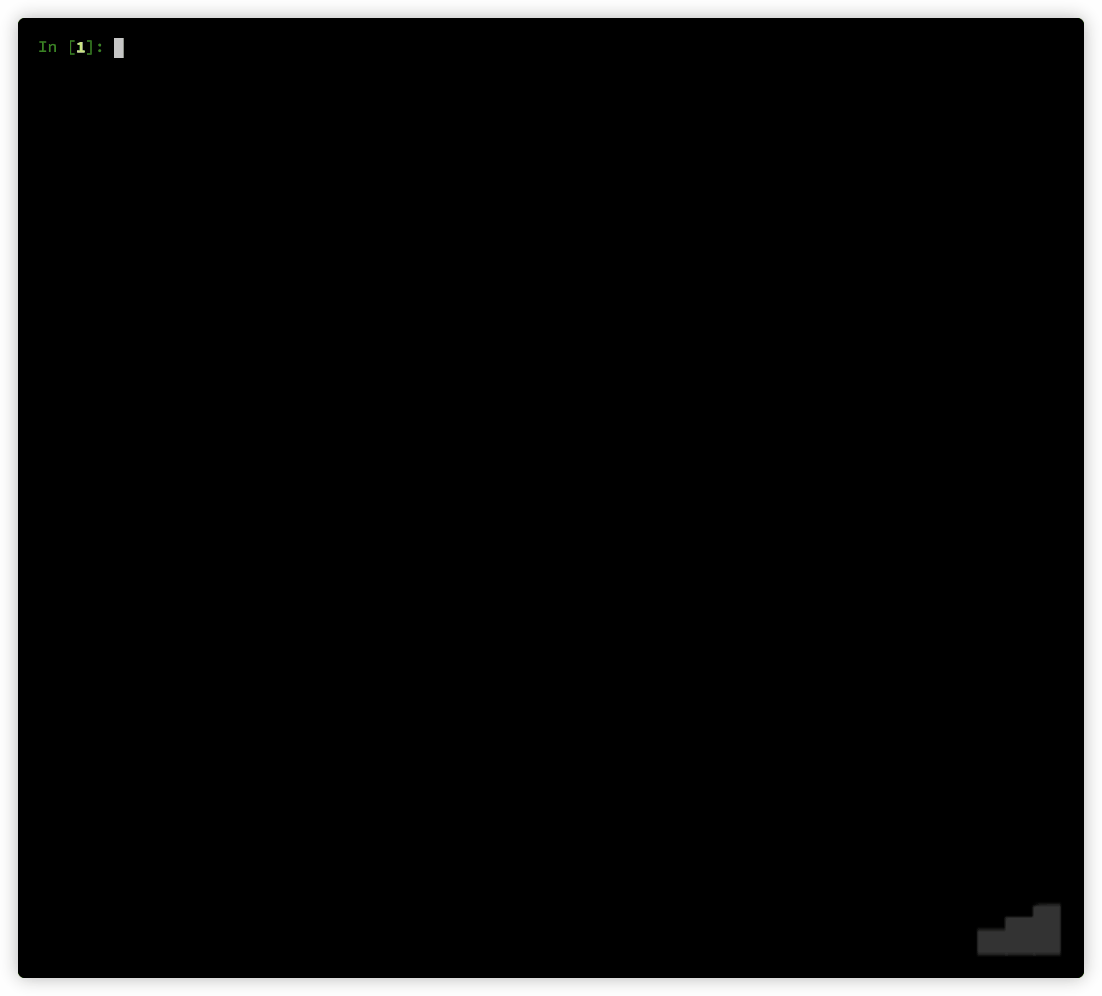
I've made these styles just to try all the animation factories I've created, but I think some of them ended up very, very cool! Use them at will, and mix them to your heart's content!
Do you want to see actual `alive-progress` bars gloriously running in your system before trying them yourself?
```sh
❯ python -m alive_progress.tools.demo
```
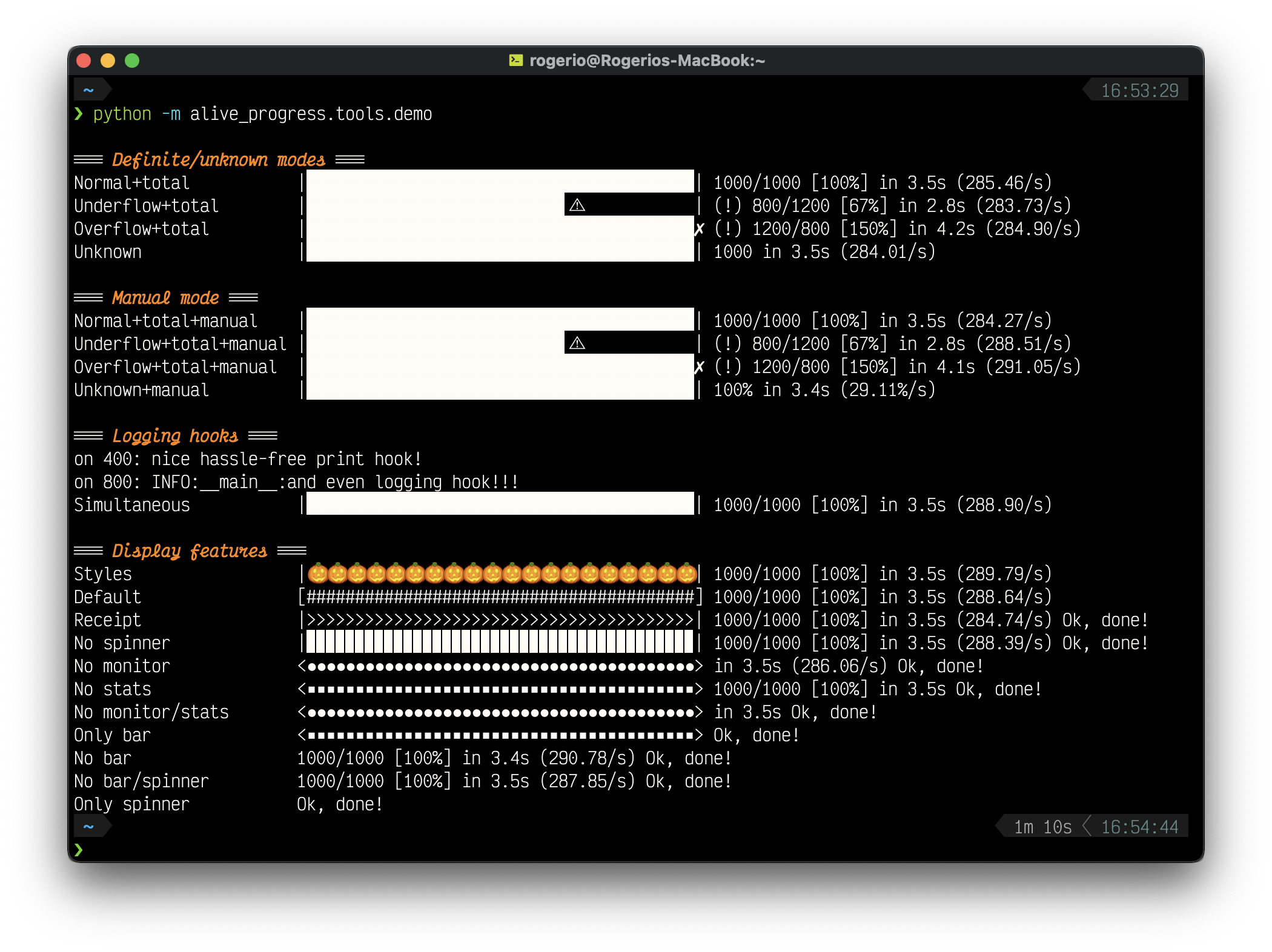
### Awake it
Cool, huh?? Now enter an `ipython` REPL and try this:
```python
from alive_progress import alive_bar
import time
for x in 1000, 1500, 700, 0:
with alive_bar(x) as bar:
for i in range(1000):
time.sleep(.005)
bar()
```
You'll see something like this, with cool animations throughout the process 😜:
```
|████████████████████████████████████████| 1000/1000 [100%] in 5.8s (171.62/s)
|██████████████████████████▋⚠︎ | (!) 1000/1500 [67%] in 5.8s (172.62/s)
|████████████████████████████████████████✗︎ (!) 1000/700 [143%] in 5.8s (172.06/s)
|████████████████████████████████████████| 1000 in 5.8s (172.45/s)
```
Nice, huh? Loved it? I knew you would, thank you 😊.
To actually use it, just wrap your normal loop in an `alive_bar` context manager like this:
```python
with alive_bar(total) as bar: # declare your expected total
for item in items: # <<-- your original loop
print(item) # process each item
bar() # call `bar()` at the end
```
And it's alive! 👏
So, in short: retrieve the items as always, enter the `alive_bar` context manager with the number of items, and then iterate/process those items, calling `bar()` at the end! It's that simple! :)
### Master it
- `items` can be any iterable, like for example, a queryset;
- the first argument of the `alive_bar` is the expected total, like `qs.count()` for querysets, `len(items)` for iterables with length, or even a static number;
- the call `bar()` is what makes the bar go forward — you usually call it in every iteration, just after finishing an item;
- if you call `bar()` too much (or too few at the end), the bar will graphically render that deviation from the expected `total`, making it very easy to notice overflows and underflows;
- to retrieve the current bar count or percentage, call `bar.current()`.
> You can get creative! Since the bar only goes forward when you call `bar()`, it is **independent of the loop**! So you can use it to monitor anything you want, like pending transactions, broken items, etc., or even call it more than once in the same iteration! So, in the end, you'll get to know how many of those "special" events there were, including their percentage relative to the total!
## Displaying messages
While inside an `alive_bar` context, you can effortlessly display messages tightly integrated with the current progress bar being displayed! It won't break in any way and will even enrich your message!
- the cool `bar.text('message')` and `bar.text = 'message'` set a situational message right within the bar, where you can display something about the current item or the phase the processing is in;
- the (📌 new) dynamic title, which can be set right at the start, but also be changed anytime with `bar.title('Title')` and `bar.title = 'Title'` — mix with `title_length` to keep the bar from changing its length;
- the usual Python `print()` statement, where `alive_bar` nicely cleans up the line, prints your message alongside the current bar position at the time, and continues the bar right below it;
- the standard Python `logging` framework, including file outputs, is also enriched exactly like the previous one;
- if you're using click CLI lib, you can even use `click.echo()` to print styled text.
Awesome right? And all of these work just the same in a terminal or in a Jupyter notebook!
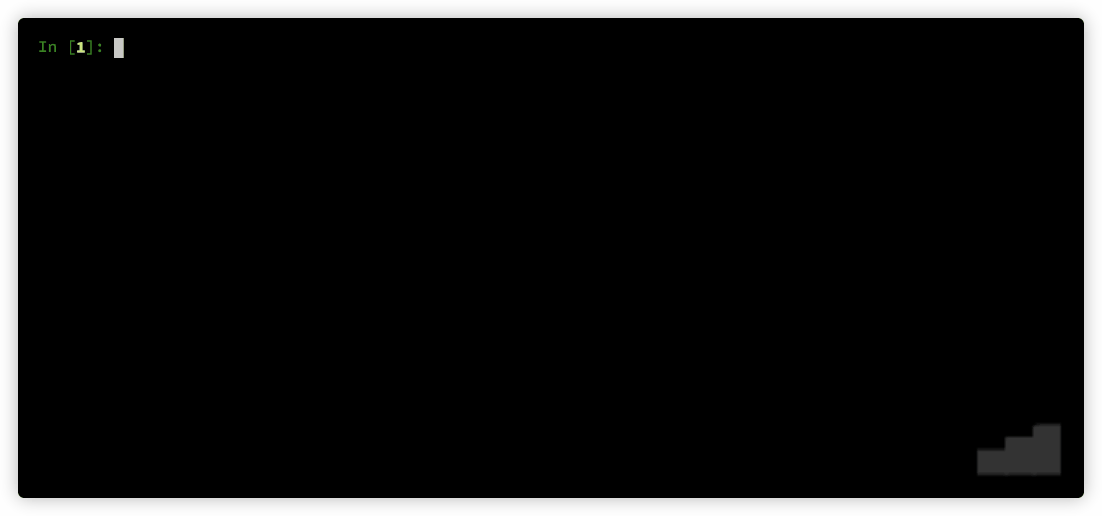
## Auto-iterating
You now have a quicker way to monitor anything! Here, the items are automatically tracked for you!
Behold the `alive_it` => the `alive_bar` iterator adapter!
Simply wrap your items with it, and loop over them as usual!
The bar will just work; it's that simple!
```python
from alive_progress import alive_it
for item in alive_it(items): # <<-- wrapped items
print(item) # process each item
```
HOW COOL IS THAT?! 😜
All `alive_bar` parameters apply but `total`, which is smarter (if not supplied, it will be auto-inferred from your data using `len` or `length_hint`), and `manual` that does not make sense here.
Note there isn't any `bar` handle at all in there. But what if you do want it, e.g. to set text messages or retrieve the current progress?
You can interact with the internal `alive_bar` by just assigning `alive_it` to a variable like this:
```python
bar = alive_it(items) # <<-- bar with wrapped items
for item in bar: # <<-- iterate on bar
print(item) # process each item
bar.text(f'ok: {item}') # WOW, it works!
```
Note that this is a slightly special `bar`, which does not support `bar()`, since the iterator adapter tracks items automatically for you. Also, it supports `finalize`, which enables you to set the title and/or text of the final receipt:
```python
alive_it(items, finalize=lambda bar: bar.text('Success!'))
```
> In a nutshell:
> - full use is always `with alive_bar() as bar`, where you iterate and call `bar()` whenever you want;
> - quick adapter use is `for item in alive_it(items)`, where items are automatically tracked;
> - full adapter use is `bar = alive_it(items)`, where in addition to items being automatically tracked, you get a special iterable `bar` able to customize the inner `alive_progress` however you want.
## Modes of operation
### Definite/unknown: Counters
Actually, the `total` argument is optional. If you do provide it, the bar enters in **definite mode**, the one used for well-bounded tasks. This mode has all the widgets `alive-progress` has to offer: progress, count, throughput, and ETA.
If you don't, the bar enters in **unknown mode**, the one used for unbounded tasks. In this mode, the whole progress bar is animated, as it's not possible to determine the progress, and therefore the ETA. But you still get the count and throughput widgets as usual.
The cool spinner is still present here alongside the progress bar, both running their animations concurrently and independently of each other, rendering a unique show in your terminal! 😜
Both definite and unknown modes use internally a **counter** to maintain progress. This is the source value which all widgets are derived from.
### Manual: Percentages
On the other hand, the **manual mode** uses internally a **percentage** to maintain progress. This enables you to get complete control of the bar position! It's usually used to monitor processes that only feed you the percentage of completion, or to generate some kind of special effects.
To use it, just include a `manual=True` argument into `alive_bar` (or `config_handler`), and you get to send any percentage to the `bar()` handler! For example, to set it to 15%, just call `bar(0.15)` — which is 15 / 100.
You can also use `total` here! If you do provide it, `alive-progress` will infer an internal _counter_ by itself, and thus will be able to offer you the same count, throughput, and ETA widgets!
If you don't, you'll at least get rough versions of the throughput and ETA widgets. The throughput will use "%/s" (percent per second), and the ETA will be till 1.0 (100%). Both are very inaccurate but better than nothing.
You can call `bar` in manual mode as frequently as you want! The refresh rate will still be asynchronously computed as usual, according to the current progress and the elapsed time, so you won't ever spam the terminal with more updates than it can handle.
### Summary of Modes
When `total` is provided all is cool:
| mode | counter | percentage | throughput | ETA | over/underflow |
|:--------:|:-------------:|:------------:|:----------:|:---:|:--------------:|
| definite | ✅ (user tick) | ✅ (inferred) | ✅ | ✅ | ✅ |
| manual | ✅ (inferred) | ✅ (user set) | ✅ | ✅ | ✅ |
When it isn't, some compromises have to be made:
| mode | counter | percentage | throughput | ETA | over/underflow |
|:-------:|:-------------:|:------------:|:------------:|:----------:|:--------------:|
| unknown | ✅ (user tick) | ❌ | ✅ | ❌ | ❌ |
| manual | ❌ | ✅ (user set) | ⚠️ (simpler) | ⚠️ (rough) | ✅ |
But actually it's quite simple, you do not need to think about which mode you should use:
Just always send the `total` if you have it, and use `manual` if you need it!
It will just work the best it can! 👏 \o/
### The `bar()` handlers
The `bar()` handlers support either relative or absolute semantics, depending on the mode:
- _definite_ and _unknown_ modes use **relative positioning**, so you can just call `bar()` to increment the counter by one, or send any other positive increment like `bar(5)` to increment by those at once;
- _manual_ modes use **absolute positioning**, so you can just call `bar(0.35)` to instantly put the bar in 35% position — this argument is mandatory here!
> The manual modes enable you to get super creative! Since you can set the bar instantly to whatever position you want, you could:
> - make it go backwards — perhaps to graphically display the timeout of something;
> - create special effects — perhaps to act as a real-time gauge of some sort.
In any case, to retrieve the current count/percentage, just call: `bar.current()`:
- in _definite_ and _unknown_ modes, this provides an **integer** — the actual internal counter;
- in _manual_ modes, this provides a **float** in the interval [0, 1] — the last percentage set.
%package -n python3-alive-progress
Summary: A new kind of Progress Bar, with real-time throughput, ETA, and very cool animations!
Provides: python-alive-progress
BuildRequires: python3-devel
BuildRequires: python3-setuptools
BuildRequires: python3-pip
%description -n python3-alive-progress
## Using `alive-progress`
### Get it
Just install with pip:
```sh
❯ pip install alive-progress
```
### Try it
If you're wondering what styles are builtin, it's `showtime`! ;)
```python
from alive_progress.styles import showtime
showtime()
```
> Note: Please disregard the path in the animated gif below, the correct one is above. These long gifs are very time-consuming to generate, so I can't make another on every single change. Thanks for your understanding.
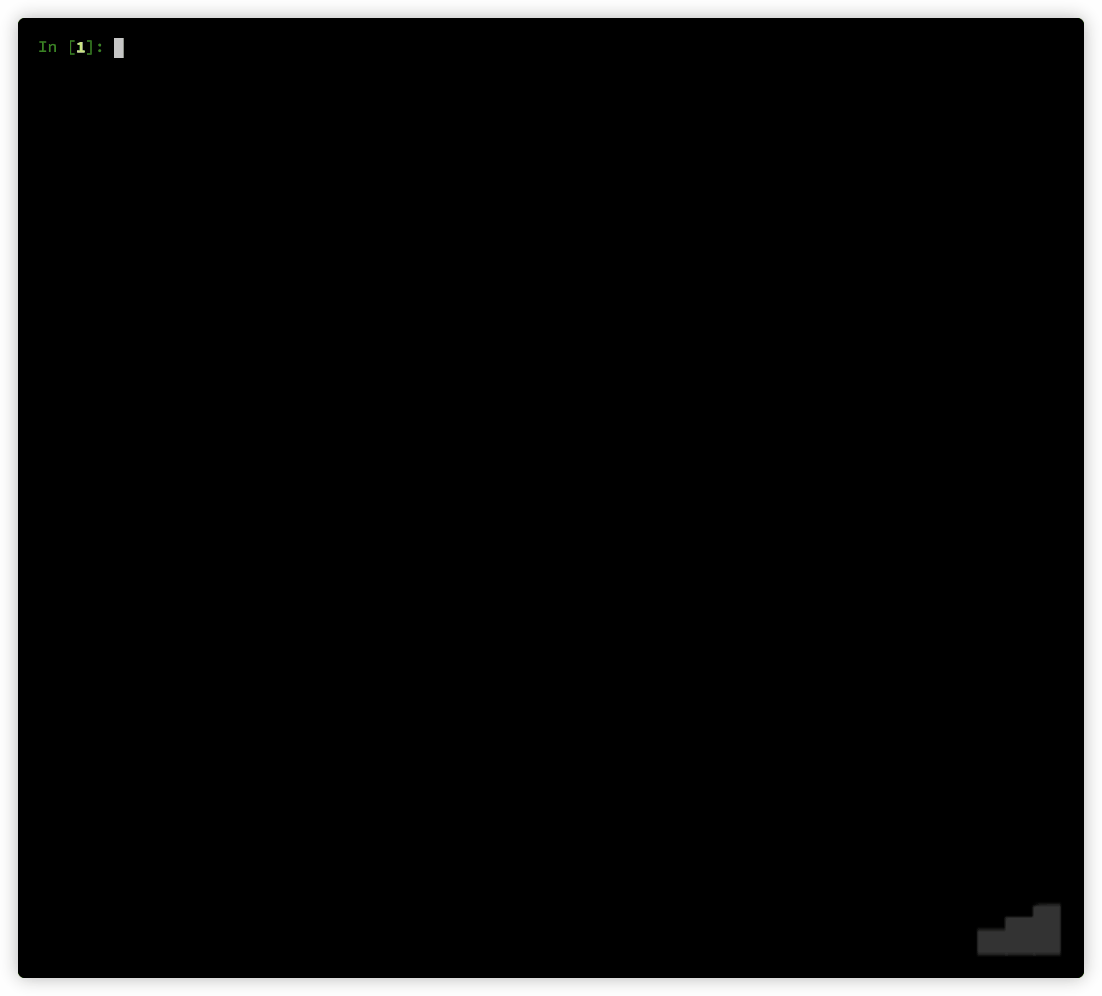
I've made these styles just to try all the animation factories I've created, but I think some of them ended up very, very cool! Use them at will, and mix them to your heart's content!
Do you want to see actual `alive-progress` bars gloriously running in your system before trying them yourself?
```sh
❯ python -m alive_progress.tools.demo
```
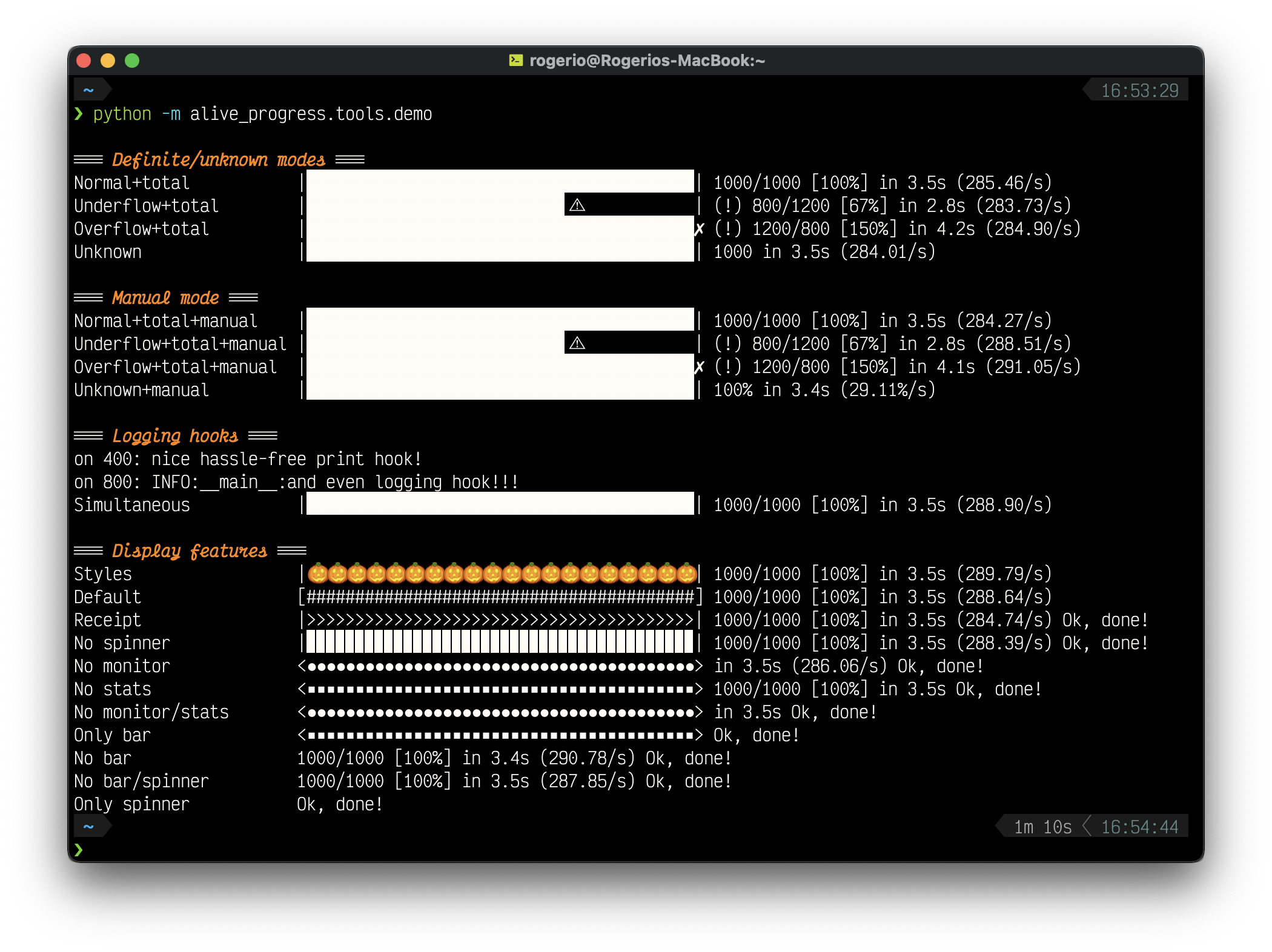
### Awake it
Cool, huh?? Now enter an `ipython` REPL and try this:
```python
from alive_progress import alive_bar
import time
for x in 1000, 1500, 700, 0:
with alive_bar(x) as bar:
for i in range(1000):
time.sleep(.005)
bar()
```
You'll see something like this, with cool animations throughout the process 😜:
```
|████████████████████████████████████████| 1000/1000 [100%] in 5.8s (171.62/s)
|██████████████████████████▋⚠︎ | (!) 1000/1500 [67%] in 5.8s (172.62/s)
|████████████████████████████████████████✗︎ (!) 1000/700 [143%] in 5.8s (172.06/s)
|████████████████████████████████████████| 1000 in 5.8s (172.45/s)
```
Nice, huh? Loved it? I knew you would, thank you 😊.
To actually use it, just wrap your normal loop in an `alive_bar` context manager like this:
```python
with alive_bar(total) as bar: # declare your expected total
for item in items: # <<-- your original loop
print(item) # process each item
bar() # call `bar()` at the end
```
And it's alive! 👏
So, in short: retrieve the items as always, enter the `alive_bar` context manager with the number of items, and then iterate/process those items, calling `bar()` at the end! It's that simple! :)
### Master it
- `items` can be any iterable, like for example, a queryset;
- the first argument of the `alive_bar` is the expected total, like `qs.count()` for querysets, `len(items)` for iterables with length, or even a static number;
- the call `bar()` is what makes the bar go forward — you usually call it in every iteration, just after finishing an item;
- if you call `bar()` too much (or too few at the end), the bar will graphically render that deviation from the expected `total`, making it very easy to notice overflows and underflows;
- to retrieve the current bar count or percentage, call `bar.current()`.
> You can get creative! Since the bar only goes forward when you call `bar()`, it is **independent of the loop**! So you can use it to monitor anything you want, like pending transactions, broken items, etc., or even call it more than once in the same iteration! So, in the end, you'll get to know how many of those "special" events there were, including their percentage relative to the total!
## Displaying messages
While inside an `alive_bar` context, you can effortlessly display messages tightly integrated with the current progress bar being displayed! It won't break in any way and will even enrich your message!
- the cool `bar.text('message')` and `bar.text = 'message'` set a situational message right within the bar, where you can display something about the current item or the phase the processing is in;
- the (📌 new) dynamic title, which can be set right at the start, but also be changed anytime with `bar.title('Title')` and `bar.title = 'Title'` — mix with `title_length` to keep the bar from changing its length;
- the usual Python `print()` statement, where `alive_bar` nicely cleans up the line, prints your message alongside the current bar position at the time, and continues the bar right below it;
- the standard Python `logging` framework, including file outputs, is also enriched exactly like the previous one;
- if you're using click CLI lib, you can even use `click.echo()` to print styled text.
Awesome right? And all of these work just the same in a terminal or in a Jupyter notebook!
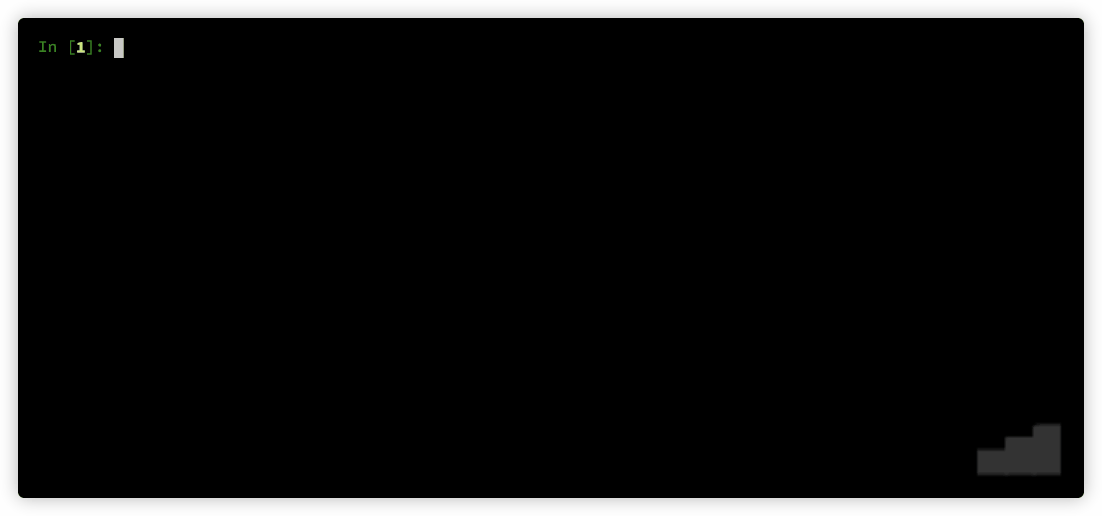
## Auto-iterating
You now have a quicker way to monitor anything! Here, the items are automatically tracked for you!
Behold the `alive_it` => the `alive_bar` iterator adapter!
Simply wrap your items with it, and loop over them as usual!
The bar will just work; it's that simple!
```python
from alive_progress import alive_it
for item in alive_it(items): # <<-- wrapped items
print(item) # process each item
```
HOW COOL IS THAT?! 😜
All `alive_bar` parameters apply but `total`, which is smarter (if not supplied, it will be auto-inferred from your data using `len` or `length_hint`), and `manual` that does not make sense here.
Note there isn't any `bar` handle at all in there. But what if you do want it, e.g. to set text messages or retrieve the current progress?
You can interact with the internal `alive_bar` by just assigning `alive_it` to a variable like this:
```python
bar = alive_it(items) # <<-- bar with wrapped items
for item in bar: # <<-- iterate on bar
print(item) # process each item
bar.text(f'ok: {item}') # WOW, it works!
```
Note that this is a slightly special `bar`, which does not support `bar()`, since the iterator adapter tracks items automatically for you. Also, it supports `finalize`, which enables you to set the title and/or text of the final receipt:
```python
alive_it(items, finalize=lambda bar: bar.text('Success!'))
```
> In a nutshell:
> - full use is always `with alive_bar() as bar`, where you iterate and call `bar()` whenever you want;
> - quick adapter use is `for item in alive_it(items)`, where items are automatically tracked;
> - full adapter use is `bar = alive_it(items)`, where in addition to items being automatically tracked, you get a special iterable `bar` able to customize the inner `alive_progress` however you want.
## Modes of operation
### Definite/unknown: Counters
Actually, the `total` argument is optional. If you do provide it, the bar enters in **definite mode**, the one used for well-bounded tasks. This mode has all the widgets `alive-progress` has to offer: progress, count, throughput, and ETA.
If you don't, the bar enters in **unknown mode**, the one used for unbounded tasks. In this mode, the whole progress bar is animated, as it's not possible to determine the progress, and therefore the ETA. But you still get the count and throughput widgets as usual.
The cool spinner is still present here alongside the progress bar, both running their animations concurrently and independently of each other, rendering a unique show in your terminal! 😜
Both definite and unknown modes use internally a **counter** to maintain progress. This is the source value which all widgets are derived from.
### Manual: Percentages
On the other hand, the **manual mode** uses internally a **percentage** to maintain progress. This enables you to get complete control of the bar position! It's usually used to monitor processes that only feed you the percentage of completion, or to generate some kind of special effects.
To use it, just include a `manual=True` argument into `alive_bar` (or `config_handler`), and you get to send any percentage to the `bar()` handler! For example, to set it to 15%, just call `bar(0.15)` — which is 15 / 100.
You can also use `total` here! If you do provide it, `alive-progress` will infer an internal _counter_ by itself, and thus will be able to offer you the same count, throughput, and ETA widgets!
If you don't, you'll at least get rough versions of the throughput and ETA widgets. The throughput will use "%/s" (percent per second), and the ETA will be till 1.0 (100%). Both are very inaccurate but better than nothing.
You can call `bar` in manual mode as frequently as you want! The refresh rate will still be asynchronously computed as usual, according to the current progress and the elapsed time, so you won't ever spam the terminal with more updates than it can handle.
### Summary of Modes
When `total` is provided all is cool:
| mode | counter | percentage | throughput | ETA | over/underflow |
|:--------:|:-------------:|:------------:|:----------:|:---:|:--------------:|
| definite | ✅ (user tick) | ✅ (inferred) | ✅ | ✅ | ✅ |
| manual | ✅ (inferred) | ✅ (user set) | ✅ | ✅ | ✅ |
When it isn't, some compromises have to be made:
| mode | counter | percentage | throughput | ETA | over/underflow |
|:-------:|:-------------:|:------------:|:------------:|:----------:|:--------------:|
| unknown | ✅ (user tick) | ❌ | ✅ | ❌ | ❌ |
| manual | ❌ | ✅ (user set) | ⚠️ (simpler) | ⚠️ (rough) | ✅ |
But actually it's quite simple, you do not need to think about which mode you should use:
Just always send the `total` if you have it, and use `manual` if you need it!
It will just work the best it can! 👏 \o/
### The `bar()` handlers
The `bar()` handlers support either relative or absolute semantics, depending on the mode:
- _definite_ and _unknown_ modes use **relative positioning**, so you can just call `bar()` to increment the counter by one, or send any other positive increment like `bar(5)` to increment by those at once;
- _manual_ modes use **absolute positioning**, so you can just call `bar(0.35)` to instantly put the bar in 35% position — this argument is mandatory here!
> The manual modes enable you to get super creative! Since you can set the bar instantly to whatever position you want, you could:
> - make it go backwards — perhaps to graphically display the timeout of something;
> - create special effects — perhaps to act as a real-time gauge of some sort.
In any case, to retrieve the current count/percentage, just call: `bar.current()`:
- in _definite_ and _unknown_ modes, this provides an **integer** — the actual internal counter;
- in _manual_ modes, this provides a **float** in the interval [0, 1] — the last percentage set.
%package help
Summary: Development documents and examples for alive-progress
Provides: python3-alive-progress-doc
%description help
## Using `alive-progress`
### Get it
Just install with pip:
```sh
❯ pip install alive-progress
```
### Try it
If you're wondering what styles are builtin, it's `showtime`! ;)
```python
from alive_progress.styles import showtime
showtime()
```
> Note: Please disregard the path in the animated gif below, the correct one is above. These long gifs are very time-consuming to generate, so I can't make another on every single change. Thanks for your understanding.
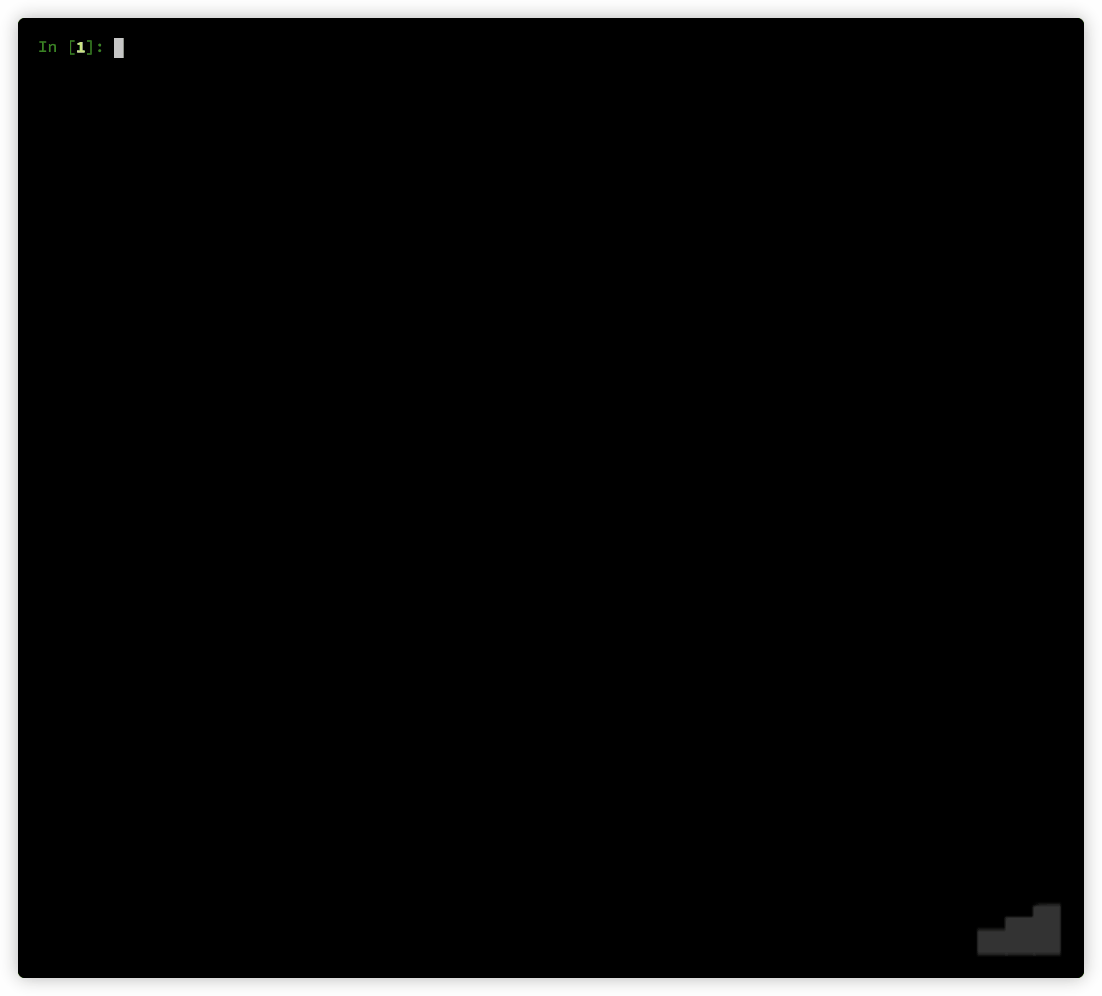
I've made these styles just to try all the animation factories I've created, but I think some of them ended up very, very cool! Use them at will, and mix them to your heart's content!
Do you want to see actual `alive-progress` bars gloriously running in your system before trying them yourself?
```sh
❯ python -m alive_progress.tools.demo
```
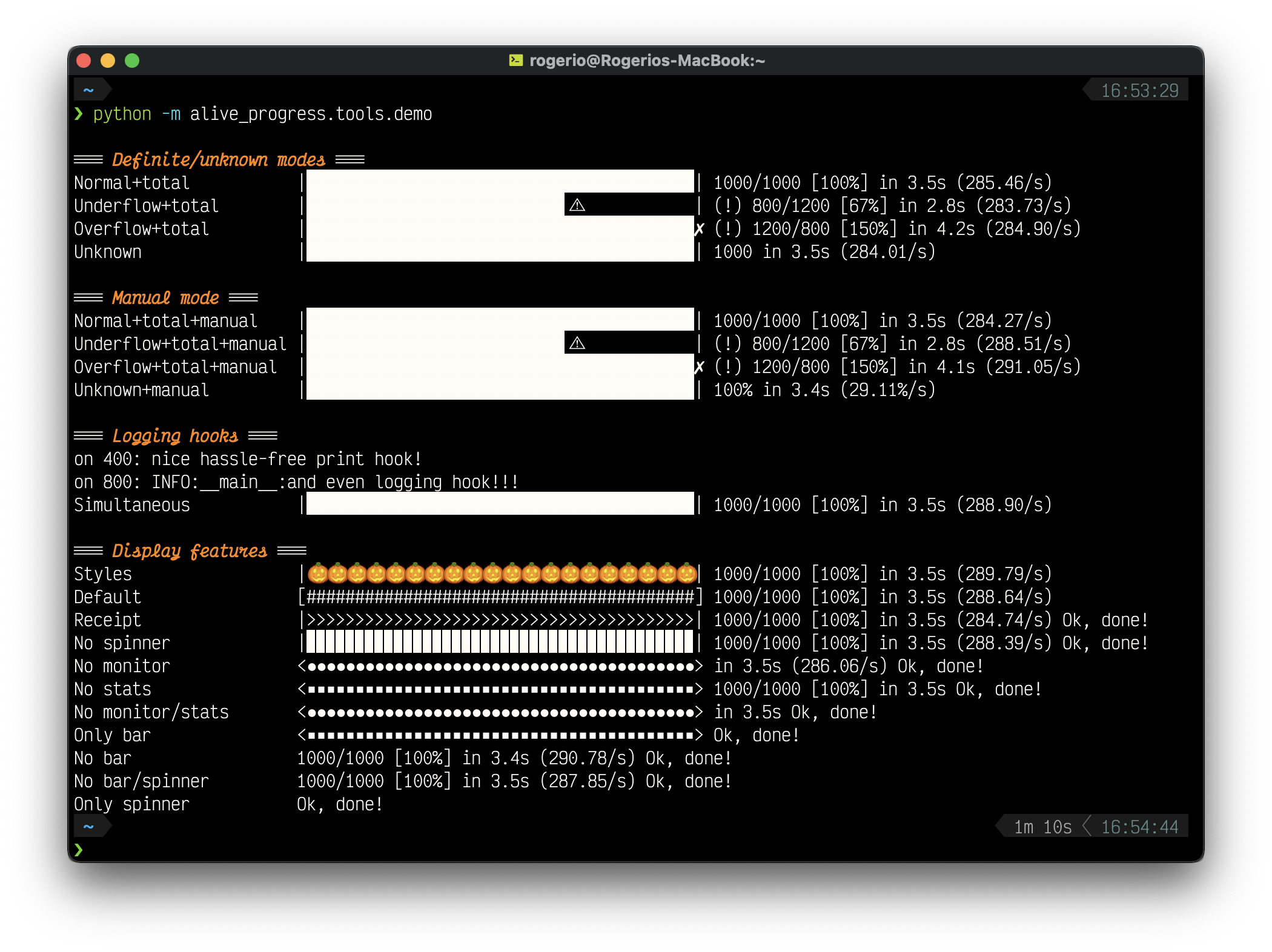
### Awake it
Cool, huh?? Now enter an `ipython` REPL and try this:
```python
from alive_progress import alive_bar
import time
for x in 1000, 1500, 700, 0:
with alive_bar(x) as bar:
for i in range(1000):
time.sleep(.005)
bar()
```
You'll see something like this, with cool animations throughout the process 😜:
```
|████████████████████████████████████████| 1000/1000 [100%] in 5.8s (171.62/s)
|██████████████████████████▋⚠︎ | (!) 1000/1500 [67%] in 5.8s (172.62/s)
|████████████████████████████████████████✗︎ (!) 1000/700 [143%] in 5.8s (172.06/s)
|████████████████████████████████████████| 1000 in 5.8s (172.45/s)
```
Nice, huh? Loved it? I knew you would, thank you 😊.
To actually use it, just wrap your normal loop in an `alive_bar` context manager like this:
```python
with alive_bar(total) as bar: # declare your expected total
for item in items: # <<-- your original loop
print(item) # process each item
bar() # call `bar()` at the end
```
And it's alive! 👏
So, in short: retrieve the items as always, enter the `alive_bar` context manager with the number of items, and then iterate/process those items, calling `bar()` at the end! It's that simple! :)
### Master it
- `items` can be any iterable, like for example, a queryset;
- the first argument of the `alive_bar` is the expected total, like `qs.count()` for querysets, `len(items)` for iterables with length, or even a static number;
- the call `bar()` is what makes the bar go forward — you usually call it in every iteration, just after finishing an item;
- if you call `bar()` too much (or too few at the end), the bar will graphically render that deviation from the expected `total`, making it very easy to notice overflows and underflows;
- to retrieve the current bar count or percentage, call `bar.current()`.
> You can get creative! Since the bar only goes forward when you call `bar()`, it is **independent of the loop**! So you can use it to monitor anything you want, like pending transactions, broken items, etc., or even call it more than once in the same iteration! So, in the end, you'll get to know how many of those "special" events there were, including their percentage relative to the total!
## Displaying messages
While inside an `alive_bar` context, you can effortlessly display messages tightly integrated with the current progress bar being displayed! It won't break in any way and will even enrich your message!
- the cool `bar.text('message')` and `bar.text = 'message'` set a situational message right within the bar, where you can display something about the current item or the phase the processing is in;
- the (📌 new) dynamic title, which can be set right at the start, but also be changed anytime with `bar.title('Title')` and `bar.title = 'Title'` — mix with `title_length` to keep the bar from changing its length;
- the usual Python `print()` statement, where `alive_bar` nicely cleans up the line, prints your message alongside the current bar position at the time, and continues the bar right below it;
- the standard Python `logging` framework, including file outputs, is also enriched exactly like the previous one;
- if you're using click CLI lib, you can even use `click.echo()` to print styled text.
Awesome right? And all of these work just the same in a terminal or in a Jupyter notebook!
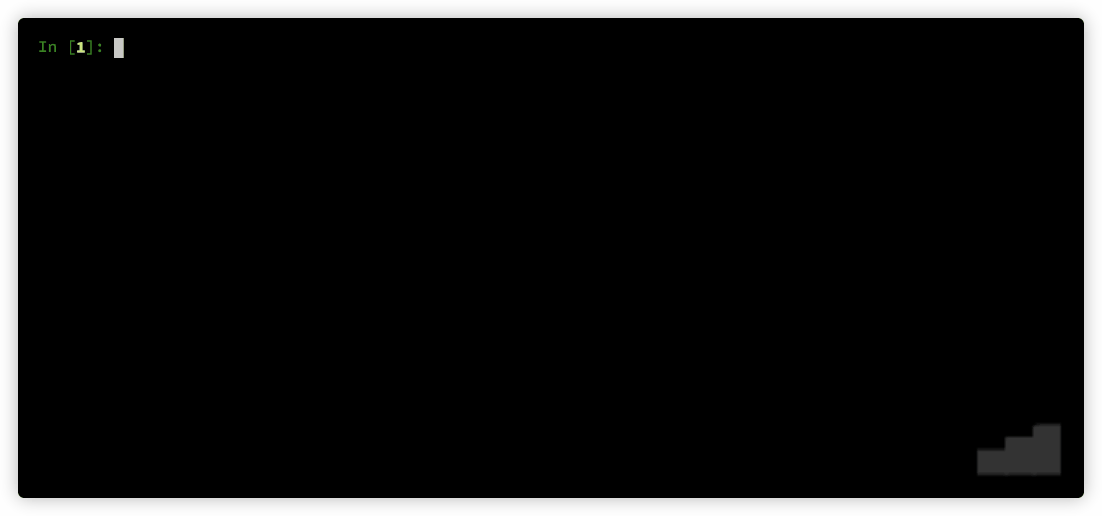
## Auto-iterating
You now have a quicker way to monitor anything! Here, the items are automatically tracked for you!
Behold the `alive_it` => the `alive_bar` iterator adapter!
Simply wrap your items with it, and loop over them as usual!
The bar will just work; it's that simple!
```python
from alive_progress import alive_it
for item in alive_it(items): # <<-- wrapped items
print(item) # process each item
```
HOW COOL IS THAT?! 😜
All `alive_bar` parameters apply but `total`, which is smarter (if not supplied, it will be auto-inferred from your data using `len` or `length_hint`), and `manual` that does not make sense here.
Note there isn't any `bar` handle at all in there. But what if you do want it, e.g. to set text messages or retrieve the current progress?
You can interact with the internal `alive_bar` by just assigning `alive_it` to a variable like this:
```python
bar = alive_it(items) # <<-- bar with wrapped items
for item in bar: # <<-- iterate on bar
print(item) # process each item
bar.text(f'ok: {item}') # WOW, it works!
```
Note that this is a slightly special `bar`, which does not support `bar()`, since the iterator adapter tracks items automatically for you. Also, it supports `finalize`, which enables you to set the title and/or text of the final receipt:
```python
alive_it(items, finalize=lambda bar: bar.text('Success!'))
```
> In a nutshell:
> - full use is always `with alive_bar() as bar`, where you iterate and call `bar()` whenever you want;
> - quick adapter use is `for item in alive_it(items)`, where items are automatically tracked;
> - full adapter use is `bar = alive_it(items)`, where in addition to items being automatically tracked, you get a special iterable `bar` able to customize the inner `alive_progress` however you want.
## Modes of operation
### Definite/unknown: Counters
Actually, the `total` argument is optional. If you do provide it, the bar enters in **definite mode**, the one used for well-bounded tasks. This mode has all the widgets `alive-progress` has to offer: progress, count, throughput, and ETA.
If you don't, the bar enters in **unknown mode**, the one used for unbounded tasks. In this mode, the whole progress bar is animated, as it's not possible to determine the progress, and therefore the ETA. But you still get the count and throughput widgets as usual.
The cool spinner is still present here alongside the progress bar, both running their animations concurrently and independently of each other, rendering a unique show in your terminal! 😜
Both definite and unknown modes use internally a **counter** to maintain progress. This is the source value which all widgets are derived from.
### Manual: Percentages
On the other hand, the **manual mode** uses internally a **percentage** to maintain progress. This enables you to get complete control of the bar position! It's usually used to monitor processes that only feed you the percentage of completion, or to generate some kind of special effects.
To use it, just include a `manual=True` argument into `alive_bar` (or `config_handler`), and you get to send any percentage to the `bar()` handler! For example, to set it to 15%, just call `bar(0.15)` — which is 15 / 100.
You can also use `total` here! If you do provide it, `alive-progress` will infer an internal _counter_ by itself, and thus will be able to offer you the same count, throughput, and ETA widgets!
If you don't, you'll at least get rough versions of the throughput and ETA widgets. The throughput will use "%/s" (percent per second), and the ETA will be till 1.0 (100%). Both are very inaccurate but better than nothing.
You can call `bar` in manual mode as frequently as you want! The refresh rate will still be asynchronously computed as usual, according to the current progress and the elapsed time, so you won't ever spam the terminal with more updates than it can handle.
### Summary of Modes
When `total` is provided all is cool:
| mode | counter | percentage | throughput | ETA | over/underflow |
|:--------:|:-------------:|:------------:|:----------:|:---:|:--------------:|
| definite | ✅ (user tick) | ✅ (inferred) | ✅ | ✅ | ✅ |
| manual | ✅ (inferred) | ✅ (user set) | ✅ | ✅ | ✅ |
When it isn't, some compromises have to be made:
| mode | counter | percentage | throughput | ETA | over/underflow |
|:-------:|:-------------:|:------------:|:------------:|:----------:|:--------------:|
| unknown | ✅ (user tick) | ❌ | ✅ | ❌ | ❌ |
| manual | ❌ | ✅ (user set) | ⚠️ (simpler) | ⚠️ (rough) | ✅ |
But actually it's quite simple, you do not need to think about which mode you should use:
Just always send the `total` if you have it, and use `manual` if you need it!
It will just work the best it can! 👏 \o/
### The `bar()` handlers
The `bar()` handlers support either relative or absolute semantics, depending on the mode:
- _definite_ and _unknown_ modes use **relative positioning**, so you can just call `bar()` to increment the counter by one, or send any other positive increment like `bar(5)` to increment by those at once;
- _manual_ modes use **absolute positioning**, so you can just call `bar(0.35)` to instantly put the bar in 35% position — this argument is mandatory here!
> The manual modes enable you to get super creative! Since you can set the bar instantly to whatever position you want, you could:
> - make it go backwards — perhaps to graphically display the timeout of something;
> - create special effects — perhaps to act as a real-time gauge of some sort.
In any case, to retrieve the current count/percentage, just call: `bar.current()`:
- in _definite_ and _unknown_ modes, this provides an **integer** — the actual internal counter;
- in _manual_ modes, this provides a **float** in the interval [0, 1] — the last percentage set.
%prep
%autosetup -n alive-progress-3.1.1
%build
%py3_build
%install
%py3_install
install -d -m755 %{buildroot}/%{_pkgdocdir}
if [ -d doc ]; then cp -arf doc %{buildroot}/%{_pkgdocdir}; fi
if [ -d docs ]; then cp -arf docs %{buildroot}/%{_pkgdocdir}; fi
if [ -d example ]; then cp -arf example %{buildroot}/%{_pkgdocdir}; fi
if [ -d examples ]; then cp -arf examples %{buildroot}/%{_pkgdocdir}; fi
pushd %{buildroot}
if [ -d usr/lib ]; then
find usr/lib -type f -printf "/%h/%f\n" >> filelist.lst
fi
if [ -d usr/lib64 ]; then
find usr/lib64 -type f -printf "/%h/%f\n" >> filelist.lst
fi
if [ -d usr/bin ]; then
find usr/bin -type f -printf "/%h/%f\n" >> filelist.lst
fi
if [ -d usr/sbin ]; then
find usr/sbin -type f -printf "/%h/%f\n" >> filelist.lst
fi
touch doclist.lst
if [ -d usr/share/man ]; then
find usr/share/man -type f -printf "/%h/%f.gz\n" >> doclist.lst
fi
popd
mv %{buildroot}/filelist.lst .
mv %{buildroot}/doclist.lst .
%files -n python3-alive-progress -f filelist.lst
%dir %{python3_sitelib}/*
%files help -f doclist.lst
%{_docdir}/*
%changelog
* Sun Apr 23 2023 Python_Bot - 3.1.1-1
- Package Spec generated