%global _empty_manifest_terminate_build 0
Name: python-Dmail
Version: 1.3.0
Release: 1
Summary: This is a simple package that provides a quick way to send emails through code.
License: MIT License
URL: https://github.com/hmiladhia/Dmail
Source0: https://mirrors.nju.edu.cn/pypi/web/packages/f9/e1/99d3de93091629ed9dcd8601c1f6df46ab468a46f2c9c3580a7412379f2e/Dmail-1.3.0.tar.gz
BuildArch: noarch
Requires: python3-markdown
Requires: python3-html2text
Requires: python3-premailer
Requires: python3-Pygments
Requires: python3-google-api-python-client
Requires: python3-google-auth-httplib2
Requires: python3-google-auth-oauthlib
%description
# Dmail
This is a simple package that provides a simple way to send emails through code.
By default, the content of the mail should be written in **markdown**
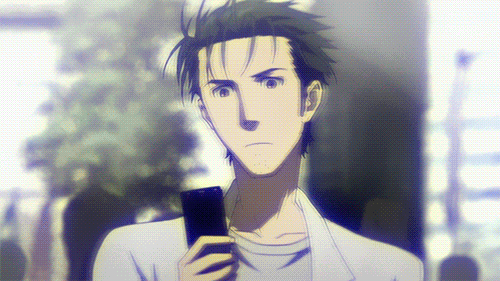
## Installation
A simple pip install will do :
```bash
python -m pip install Dmail
```
If you want support for code highlighting with pygments:
```bash
python -m pip install Dmail[CodeHighlight]
```
## How to use:
```python
import os
from Dmail.esp import Gmail
# email info
recipient_email = "xxx@gmail.com"
cc_email = "yyy@hotmail.fr"
sender_email = os.environ.get('email')
password = os.environ.get('password')
# Send Markdown e-mails :
message = """
# Email Content
This is a **test**

| Collumn1 | Collumn2 | Collumn3 |
| :------: | :------- | -------- |
| Content1 | Content2 | Content3 |
this is some other text
[^1]: This is a footnote.
[^2]: This is another footnote.
"""
with Gmail(sender_email, password) as gmail:
gmail.send(message, recipient_email, subject="[Dmail] Markdown Demo", cc=cc_email,
attachments=[r"path\to\image.jpg", r'path\to\pdf.pdf', r'path\to\text.txt'])
```
- You can send an e-mail loaded from a file:
```python
with Gmail(sender_email, password) as gmail:
gmail.send_from_file(r"path\to\my_message.md", recipient_email,
subject="[Dmail] Markdown File")
```
- You can also send *text* or *html* content by specifying the **subtype** :
```python
from Dmail.esp import Hotmail
message = "Simple e-mail"
with Hotmail(sender_email, password) as hotmail:
hotmail.add_attachments(r"path\to\image.jpg", "another_name.jpg")
hotmail.send(message, recipient_email, "[Dmail] Text demo", subtype='text')
```
- The usage of a custom **CSS stylesheet** is possible :
```python
with Hotmail(sender_email, password, styles=r'path\to\style.css') as mail:
mail.send(message, recipient_email, subject="[Dmail] Markdown Style")
```
### Custom SMTP Server
- You can use a custom smtp server and port:
```python
from Dmail import Email
with Email(mail_server, mail_port, sender_email, password) as email:
email.send(message, recipient_email, "[Dmail] Text demo")
```
### APIs
#### Gmail Api
##### Installation
To use the Gmail Api you need to install extra packages :
```bash
python -m pip install Dmail[GmailApi]
```
##### First use
You can also use the **Gmail API** through a token !
You'll need to download *"credentials.json"* ( Step 1 of this guide : https://developers.google.com/gmail/api/quickstart/python#step_1_turn_on_the )
##### Send Email
```python
from Dmail.api import GmailApi
message = """
# Email Content
This is a **test**
"""
with GmailApi(sender_email, 'token.pickle', 'credentials.json') as email:
email.send(message, recipient_email, subject='[Dmail] Gmail Api - test')
```
Once you've given the rights, this will create the "*token.pickle*" that you can use later !
##### Create draft
Instead of sending the email, you can create it as a draft
```python
from Dmail.api import GmailApi
with GmailApi(sender_email, 'token.pickle') as email:
email.create_draft(message, recipient_email, '[Dmail] Gmail Api - draft')
```
If you like the project and want to support us, you can buy us a coffee here:
%package -n python3-Dmail
Summary: This is a simple package that provides a quick way to send emails through code.
Provides: python-Dmail
BuildRequires: python3-devel
BuildRequires: python3-setuptools
BuildRequires: python3-pip
%description -n python3-Dmail
# Dmail
This is a simple package that provides a simple way to send emails through code.
By default, the content of the mail should be written in **markdown**
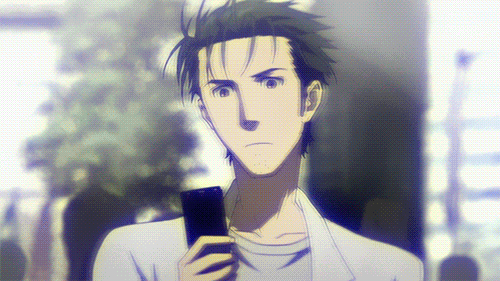
## Installation
A simple pip install will do :
```bash
python -m pip install Dmail
```
If you want support for code highlighting with pygments:
```bash
python -m pip install Dmail[CodeHighlight]
```
## How to use:
```python
import os
from Dmail.esp import Gmail
# email info
recipient_email = "xxx@gmail.com"
cc_email = "yyy@hotmail.fr"
sender_email = os.environ.get('email')
password = os.environ.get('password')
# Send Markdown e-mails :
message = """
# Email Content
This is a **test**

| Collumn1 | Collumn2 | Collumn3 |
| :------: | :------- | -------- |
| Content1 | Content2 | Content3 |
this is some other text
[^1]: This is a footnote.
[^2]: This is another footnote.
"""
with Gmail(sender_email, password) as gmail:
gmail.send(message, recipient_email, subject="[Dmail] Markdown Demo", cc=cc_email,
attachments=[r"path\to\image.jpg", r'path\to\pdf.pdf', r'path\to\text.txt'])
```
- You can send an e-mail loaded from a file:
```python
with Gmail(sender_email, password) as gmail:
gmail.send_from_file(r"path\to\my_message.md", recipient_email,
subject="[Dmail] Markdown File")
```
- You can also send *text* or *html* content by specifying the **subtype** :
```python
from Dmail.esp import Hotmail
message = "Simple e-mail"
with Hotmail(sender_email, password) as hotmail:
hotmail.add_attachments(r"path\to\image.jpg", "another_name.jpg")
hotmail.send(message, recipient_email, "[Dmail] Text demo", subtype='text')
```
- The usage of a custom **CSS stylesheet** is possible :
```python
with Hotmail(sender_email, password, styles=r'path\to\style.css') as mail:
mail.send(message, recipient_email, subject="[Dmail] Markdown Style")
```
### Custom SMTP Server
- You can use a custom smtp server and port:
```python
from Dmail import Email
with Email(mail_server, mail_port, sender_email, password) as email:
email.send(message, recipient_email, "[Dmail] Text demo")
```
### APIs
#### Gmail Api
##### Installation
To use the Gmail Api you need to install extra packages :
```bash
python -m pip install Dmail[GmailApi]
```
##### First use
You can also use the **Gmail API** through a token !
You'll need to download *"credentials.json"* ( Step 1 of this guide : https://developers.google.com/gmail/api/quickstart/python#step_1_turn_on_the )
##### Send Email
```python
from Dmail.api import GmailApi
message = """
# Email Content
This is a **test**
"""
with GmailApi(sender_email, 'token.pickle', 'credentials.json') as email:
email.send(message, recipient_email, subject='[Dmail] Gmail Api - test')
```
Once you've given the rights, this will create the "*token.pickle*" that you can use later !
##### Create draft
Instead of sending the email, you can create it as a draft
```python
from Dmail.api import GmailApi
with GmailApi(sender_email, 'token.pickle') as email:
email.create_draft(message, recipient_email, '[Dmail] Gmail Api - draft')
```
If you like the project and want to support us, you can buy us a coffee here:
%package help
Summary: Development documents and examples for Dmail
Provides: python3-Dmail-doc
%description help
# Dmail
This is a simple package that provides a simple way to send emails through code.
By default, the content of the mail should be written in **markdown**
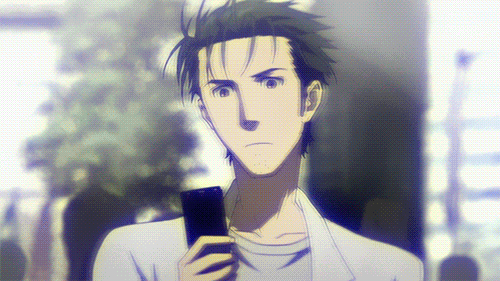
## Installation
A simple pip install will do :
```bash
python -m pip install Dmail
```
If you want support for code highlighting with pygments:
```bash
python -m pip install Dmail[CodeHighlight]
```
## How to use:
```python
import os
from Dmail.esp import Gmail
# email info
recipient_email = "xxx@gmail.com"
cc_email = "yyy@hotmail.fr"
sender_email = os.environ.get('email')
password = os.environ.get('password')
# Send Markdown e-mails :
message = """
# Email Content
This is a **test**

| Collumn1 | Collumn2 | Collumn3 |
| :------: | :------- | -------- |
| Content1 | Content2 | Content3 |
this is some other text
[^1]: This is a footnote.
[^2]: This is another footnote.
"""
with Gmail(sender_email, password) as gmail:
gmail.send(message, recipient_email, subject="[Dmail] Markdown Demo", cc=cc_email,
attachments=[r"path\to\image.jpg", r'path\to\pdf.pdf', r'path\to\text.txt'])
```
- You can send an e-mail loaded from a file:
```python
with Gmail(sender_email, password) as gmail:
gmail.send_from_file(r"path\to\my_message.md", recipient_email,
subject="[Dmail] Markdown File")
```
- You can also send *text* or *html* content by specifying the **subtype** :
```python
from Dmail.esp import Hotmail
message = "Simple e-mail"
with Hotmail(sender_email, password) as hotmail:
hotmail.add_attachments(r"path\to\image.jpg", "another_name.jpg")
hotmail.send(message, recipient_email, "[Dmail] Text demo", subtype='text')
```
- The usage of a custom **CSS stylesheet** is possible :
```python
with Hotmail(sender_email, password, styles=r'path\to\style.css') as mail:
mail.send(message, recipient_email, subject="[Dmail] Markdown Style")
```
### Custom SMTP Server
- You can use a custom smtp server and port:
```python
from Dmail import Email
with Email(mail_server, mail_port, sender_email, password) as email:
email.send(message, recipient_email, "[Dmail] Text demo")
```
### APIs
#### Gmail Api
##### Installation
To use the Gmail Api you need to install extra packages :
```bash
python -m pip install Dmail[GmailApi]
```
##### First use
You can also use the **Gmail API** through a token !
You'll need to download *"credentials.json"* ( Step 1 of this guide : https://developers.google.com/gmail/api/quickstart/python#step_1_turn_on_the )
##### Send Email
```python
from Dmail.api import GmailApi
message = """
# Email Content
This is a **test**
"""
with GmailApi(sender_email, 'token.pickle', 'credentials.json') as email:
email.send(message, recipient_email, subject='[Dmail] Gmail Api - test')
```
Once you've given the rights, this will create the "*token.pickle*" that you can use later !
##### Create draft
Instead of sending the email, you can create it as a draft
```python
from Dmail.api import GmailApi
with GmailApi(sender_email, 'token.pickle') as email:
email.create_draft(message, recipient_email, '[Dmail] Gmail Api - draft')
```
If you like the project and want to support us, you can buy us a coffee here:
%prep
%autosetup -n Dmail-1.3.0
%build
%py3_build
%install
%py3_install
install -d -m755 %{buildroot}/%{_pkgdocdir}
if [ -d doc ]; then cp -arf doc %{buildroot}/%{_pkgdocdir}; fi
if [ -d docs ]; then cp -arf docs %{buildroot}/%{_pkgdocdir}; fi
if [ -d example ]; then cp -arf example %{buildroot}/%{_pkgdocdir}; fi
if [ -d examples ]; then cp -arf examples %{buildroot}/%{_pkgdocdir}; fi
pushd %{buildroot}
if [ -d usr/lib ]; then
find usr/lib -type f -printf "/%h/%f\n" >> filelist.lst
fi
if [ -d usr/lib64 ]; then
find usr/lib64 -type f -printf "/%h/%f\n" >> filelist.lst
fi
if [ -d usr/bin ]; then
find usr/bin -type f -printf "/%h/%f\n" >> filelist.lst
fi
if [ -d usr/sbin ]; then
find usr/sbin -type f -printf "/%h/%f\n" >> filelist.lst
fi
touch doclist.lst
if [ -d usr/share/man ]; then
find usr/share/man -type f -printf "/%h/%f.gz\n" >> doclist.lst
fi
popd
mv %{buildroot}/filelist.lst .
mv %{buildroot}/doclist.lst .
%files -n python3-Dmail -f filelist.lst
%dir %{python3_sitelib}/*
%files help -f doclist.lst
%{_docdir}/*
%changelog
* Fri May 05 2023 Python_Bot - 1.3.0-1
- Package Spec generated