%global _empty_manifest_terminate_build 0
Name: python-tkinter-tooltip
Version: 2.1.0
Release: 1
Summary: An easy and customisable ToolTip implementation for Tkinter
License: MIT
URL: https://github.com/gnikit/tkinter-tooltip
Source0: https://mirrors.nju.edu.cn/pypi/web/packages/af/ed/4d236a87382ba0ac28a4dfd9551ffec8b2b76111fd416187680a3e352472/tkinter-tooltip-2.1.0.tar.gz
BuildArch: noarch
Requires: python3-importlib-metadata
Requires: python3-typing-extensions
Requires: python3-pytest
Requires: python3-pytest-cov
Requires: python3-black
Requires: python3-isort
Requires: python3-sphinx
Requires: python3-sphinx-autodoc-typehints
Requires: python3-sphinx-rtd-theme
Requires: python3-sphinxprettysearchresults
Requires: python3-myst-parser
Requires: python3-docutils
%description
[](https://pepy.tech/project/tkinter-tooltip)
[](https://pypi.org/project/tkinter-tooltip/)
[](https://github.com/gnikit/tkinter-tooltip/actions/workflows/python-publish.yml)
[](https://github.com/gnikit/tkinter-tooltip/actions/workflows/docs.yml)
[](https://www.codefactor.io/repository/github/gnikit/tkinter-tooltip)
[](https://github.com/gnikit/tkinter-tooltip/blob/master/LICENSE)
[](https://github.com/psf/black)
[](https://github.com/sponsors/gnikit)
[](https://paypal.me/inikit)
[](https://www.buymeacoffee.com/gnikit)
# tkinter-tooltip
## What this is
This is a simple yet fully customisable tooltip/pop-up implementation for
`tkinter` widgets. It is capable of fully integrating with custom `tkinter`
themes both light and dark ones.

## Features
- normal tooltips
- show tooltip with `s` seconds `delay`
- tooltip tracks mouse cursor
- tooltip displays strings and string returning functions
- fully customisable, tooltip inherits underlying theme style
## Install
```shell
pip install tkinter-tooltip
```
## Examples
### Normal tooltips
By default the tooltip activates when entering and/or moving in the widget are
and deactivates when leaving and/or pressing any button.
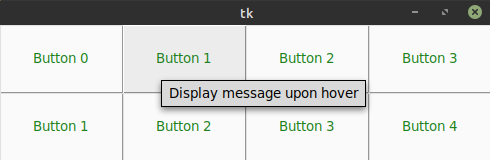
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
ToolTip(b, msg="Hover info")
app.mainloop()
```
### Delayed tooltip
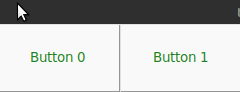
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
ToolTip(b, msg="Hover info", delay=2.0) # True by default
app.mainloop()
```
### Tracking tooltip
Have the tooltip follow the mousse cursor around when moving.
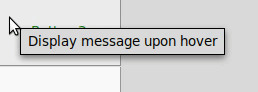
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
ToolTip(b, msg="Hover info", follow=True) # True by default
app.mainloop()
```
### Function as tooltip
Here the tooltip returns the value of `time.asctime()` which updates with every
movement. You can control the refresh rate of the `ToolTip` through the `refresh`
argument by default it is set to `1s`.
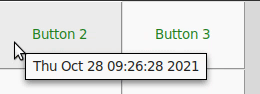
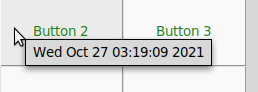
```python
import time
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
# NOTE: pass the function itself not the return value
ToolTip(b, msg=time.asctime, delay=0)
app.mainloop()
```
### Themed tooltip
`tkinter-tooltip` is fully aware of the underlying theme (in this case a dark theme),
and can even be furher customised by passing `tk` styling arguments to the tooltip
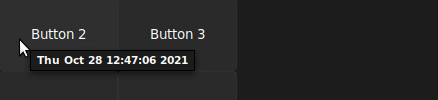
Style tooltip and underlying the button. If a full theme has been used then
the `ToolTip` will inherit the settings of the theme by default.
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
s = ttk.Style()
s.configure("custom.TButton", foreground="#ffffff", background="#1c1c1c")
b = ttk.Button(app, text="Button", style="custom.TButton")
b.pack()
ToolTip(b, msg="Hover info", delay=0,
parent_kwargs={"bg": "black", "padx": 5, "pady": 5},
fg="#ffffff", bg="#1c1c1c", padx=10, pady=10)
app.mainloop()
```
## Acknowledgements
`tkinter-tooltip` is based on the original work performed by
[Tucker Beck](http://code.activestate.com/recipes/576688-tooltip-for-tkinter/)
licensed under an MIT License.
## License
MIT License
%package -n python3-tkinter-tooltip
Summary: An easy and customisable ToolTip implementation for Tkinter
Provides: python-tkinter-tooltip
BuildRequires: python3-devel
BuildRequires: python3-setuptools
BuildRequires: python3-pip
%description -n python3-tkinter-tooltip
[](https://pepy.tech/project/tkinter-tooltip)
[](https://pypi.org/project/tkinter-tooltip/)
[](https://github.com/gnikit/tkinter-tooltip/actions/workflows/python-publish.yml)
[](https://github.com/gnikit/tkinter-tooltip/actions/workflows/docs.yml)
[](https://www.codefactor.io/repository/github/gnikit/tkinter-tooltip)
[](https://github.com/gnikit/tkinter-tooltip/blob/master/LICENSE)
[](https://github.com/psf/black)
[](https://github.com/sponsors/gnikit)
[](https://paypal.me/inikit)
[](https://www.buymeacoffee.com/gnikit)
# tkinter-tooltip
## What this is
This is a simple yet fully customisable tooltip/pop-up implementation for
`tkinter` widgets. It is capable of fully integrating with custom `tkinter`
themes both light and dark ones.

## Features
- normal tooltips
- show tooltip with `s` seconds `delay`
- tooltip tracks mouse cursor
- tooltip displays strings and string returning functions
- fully customisable, tooltip inherits underlying theme style
## Install
```shell
pip install tkinter-tooltip
```
## Examples
### Normal tooltips
By default the tooltip activates when entering and/or moving in the widget are
and deactivates when leaving and/or pressing any button.
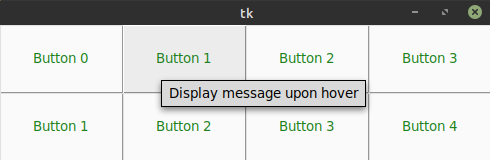
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
ToolTip(b, msg="Hover info")
app.mainloop()
```
### Delayed tooltip
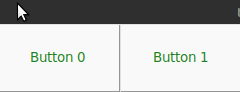
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
ToolTip(b, msg="Hover info", delay=2.0) # True by default
app.mainloop()
```
### Tracking tooltip
Have the tooltip follow the mousse cursor around when moving.
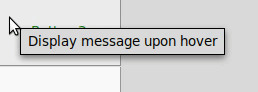
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
ToolTip(b, msg="Hover info", follow=True) # True by default
app.mainloop()
```
### Function as tooltip
Here the tooltip returns the value of `time.asctime()` which updates with every
movement. You can control the refresh rate of the `ToolTip` through the `refresh`
argument by default it is set to `1s`.
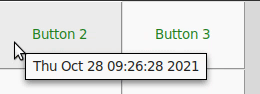
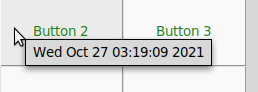
```python
import time
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
# NOTE: pass the function itself not the return value
ToolTip(b, msg=time.asctime, delay=0)
app.mainloop()
```
### Themed tooltip
`tkinter-tooltip` is fully aware of the underlying theme (in this case a dark theme),
and can even be furher customised by passing `tk` styling arguments to the tooltip
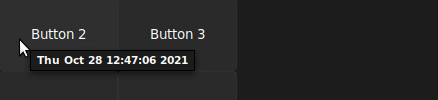
Style tooltip and underlying the button. If a full theme has been used then
the `ToolTip` will inherit the settings of the theme by default.
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
s = ttk.Style()
s.configure("custom.TButton", foreground="#ffffff", background="#1c1c1c")
b = ttk.Button(app, text="Button", style="custom.TButton")
b.pack()
ToolTip(b, msg="Hover info", delay=0,
parent_kwargs={"bg": "black", "padx": 5, "pady": 5},
fg="#ffffff", bg="#1c1c1c", padx=10, pady=10)
app.mainloop()
```
## Acknowledgements
`tkinter-tooltip` is based on the original work performed by
[Tucker Beck](http://code.activestate.com/recipes/576688-tooltip-for-tkinter/)
licensed under an MIT License.
## License
MIT License
%package help
Summary: Development documents and examples for tkinter-tooltip
Provides: python3-tkinter-tooltip-doc
%description help
[](https://pepy.tech/project/tkinter-tooltip)
[](https://pypi.org/project/tkinter-tooltip/)
[](https://github.com/gnikit/tkinter-tooltip/actions/workflows/python-publish.yml)
[](https://github.com/gnikit/tkinter-tooltip/actions/workflows/docs.yml)
[](https://www.codefactor.io/repository/github/gnikit/tkinter-tooltip)
[](https://github.com/gnikit/tkinter-tooltip/blob/master/LICENSE)
[](https://github.com/psf/black)
[](https://github.com/sponsors/gnikit)
[](https://paypal.me/inikit)
[](https://www.buymeacoffee.com/gnikit)
# tkinter-tooltip
## What this is
This is a simple yet fully customisable tooltip/pop-up implementation for
`tkinter` widgets. It is capable of fully integrating with custom `tkinter`
themes both light and dark ones.

## Features
- normal tooltips
- show tooltip with `s` seconds `delay`
- tooltip tracks mouse cursor
- tooltip displays strings and string returning functions
- fully customisable, tooltip inherits underlying theme style
## Install
```shell
pip install tkinter-tooltip
```
## Examples
### Normal tooltips
By default the tooltip activates when entering and/or moving in the widget are
and deactivates when leaving and/or pressing any button.
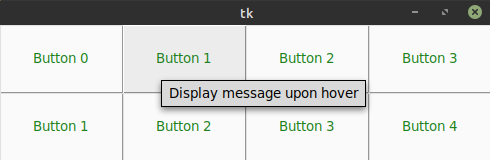
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
ToolTip(b, msg="Hover info")
app.mainloop()
```
### Delayed tooltip
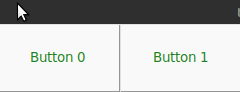
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
ToolTip(b, msg="Hover info", delay=2.0) # True by default
app.mainloop()
```
### Tracking tooltip
Have the tooltip follow the mousse cursor around when moving.
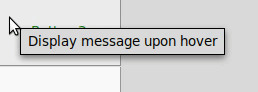
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
ToolTip(b, msg="Hover info", follow=True) # True by default
app.mainloop()
```
### Function as tooltip
Here the tooltip returns the value of `time.asctime()` which updates with every
movement. You can control the refresh rate of the `ToolTip` through the `refresh`
argument by default it is set to `1s`.
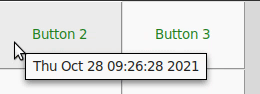
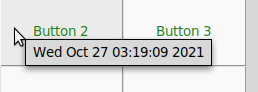
```python
import time
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
b = ttk.Button(app, text="Button")
b.pack()
# NOTE: pass the function itself not the return value
ToolTip(b, msg=time.asctime, delay=0)
app.mainloop()
```
### Themed tooltip
`tkinter-tooltip` is fully aware of the underlying theme (in this case a dark theme),
and can even be furher customised by passing `tk` styling arguments to the tooltip
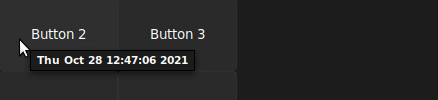
Style tooltip and underlying the button. If a full theme has been used then
the `ToolTip` will inherit the settings of the theme by default.
```python
import tkinter as tk
import tkinter.ttk as ttk
from tktooltip import ToolTip
app = tk.Tk()
s = ttk.Style()
s.configure("custom.TButton", foreground="#ffffff", background="#1c1c1c")
b = ttk.Button(app, text="Button", style="custom.TButton")
b.pack()
ToolTip(b, msg="Hover info", delay=0,
parent_kwargs={"bg": "black", "padx": 5, "pady": 5},
fg="#ffffff", bg="#1c1c1c", padx=10, pady=10)
app.mainloop()
```
## Acknowledgements
`tkinter-tooltip` is based on the original work performed by
[Tucker Beck](http://code.activestate.com/recipes/576688-tooltip-for-tkinter/)
licensed under an MIT License.
## License
MIT License
%prep
%autosetup -n tkinter-tooltip-2.1.0
%build
%py3_build
%install
%py3_install
install -d -m755 %{buildroot}/%{_pkgdocdir}
if [ -d doc ]; then cp -arf doc %{buildroot}/%{_pkgdocdir}; fi
if [ -d docs ]; then cp -arf docs %{buildroot}/%{_pkgdocdir}; fi
if [ -d example ]; then cp -arf example %{buildroot}/%{_pkgdocdir}; fi
if [ -d examples ]; then cp -arf examples %{buildroot}/%{_pkgdocdir}; fi
pushd %{buildroot}
if [ -d usr/lib ]; then
find usr/lib -type f -printf "/%h/%f\n" >> filelist.lst
fi
if [ -d usr/lib64 ]; then
find usr/lib64 -type f -printf "/%h/%f\n" >> filelist.lst
fi
if [ -d usr/bin ]; then
find usr/bin -type f -printf "/%h/%f\n" >> filelist.lst
fi
if [ -d usr/sbin ]; then
find usr/sbin -type f -printf "/%h/%f\n" >> filelist.lst
fi
touch doclist.lst
if [ -d usr/share/man ]; then
find usr/share/man -type f -printf "/%h/%f.gz\n" >> doclist.lst
fi
popd
mv %{buildroot}/filelist.lst .
mv %{buildroot}/doclist.lst .
%files -n python3-tkinter-tooltip -f filelist.lst
%dir %{python3_sitelib}/*
%files help -f doclist.lst
%{_docdir}/*
%changelog
* Wed May 10 2023 Python_Bot - 2.1.0-1
- Package Spec generated