%global _empty_manifest_terminate_build 0
Name: python-bimpy
Version: 0.1.1
Release: 1
Summary: bimpy - bundled imgui for python
License: MIT
URL: https://github.com/podgorskiy/bimpy
Source0: https://mirrors.nju.edu.cn/pypi/web/packages/59/10/fa47d72afbea9205804b96f3f13f56f6da0297837c05305d44776c3ebdf8/bimpy-0.1.1.tar.gz
%description
Core API | Using `bimpy.App` class |
```python import bimpy as bp ctx = bp.Context() ctx.init(600, 600, "Hello") s = bp.String() f = bp.Float() while not ctx.should_close(): with ctx: bp.text("Hello, world!") if bp.button("OK"): print(s.value) bp.input_text('string', str, 256) bp.slider_float("float", f, 0, 1) ``` | ```python import bimpy as bp class App(bp.App): def __init__(self): super(App, self).__init__(title='Test') self.s = bp.String() self.f = bp.Float() def on_update(self): bp.text("Hello, world!") if bp.button("OK"): print(self.s.value) bp.input_text('string', self.s, 256) bp.slider_float("float", self.f, 0, 1) app = App() app.run() ``` |
```python import bimpy from PIL import Image ctx = bimpy.Context() ctx.init(800, 800, "Image") image = Image.open("test.png") im = bimpy.Image(image) while not ctx.should_close(): with ctx: bimpy.text("Display PIL Image") bimpy.image(im) ``` | 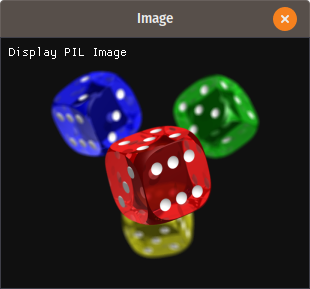 |
```python import bimpy from PIL import Image import numpy as np ctx = bimpy.Context() ctx.init(800, 800, "Image") image = np.asarray(Image.open("3.png"), dtype=np.uint8) im = bimpy.Image(image) while not ctx.should_close(): with ctx: bimpy.text("Display Image of type:") bimpy.same_line() bimpy.text(str(type(image))) bimpy.image(im) ``` | 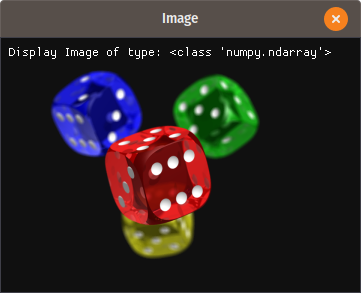 |
Core API | Using `bimpy.App` class |
```python import bimpy as bp ctx = bp.Context() ctx.init(600, 600, "Hello") s = bp.String() f = bp.Float() while not ctx.should_close(): with ctx: bp.text("Hello, world!") if bp.button("OK"): print(s.value) bp.input_text('string', str, 256) bp.slider_float("float", f, 0, 1) ``` | ```python import bimpy as bp class App(bp.App): def __init__(self): super(App, self).__init__(title='Test') self.s = bp.String() self.f = bp.Float() def on_update(self): bp.text("Hello, world!") if bp.button("OK"): print(self.s.value) bp.input_text('string', self.s, 256) bp.slider_float("float", self.f, 0, 1) app = App() app.run() ``` |
```python import bimpy from PIL import Image ctx = bimpy.Context() ctx.init(800, 800, "Image") image = Image.open("test.png") im = bimpy.Image(image) while not ctx.should_close(): with ctx: bimpy.text("Display PIL Image") bimpy.image(im) ``` | 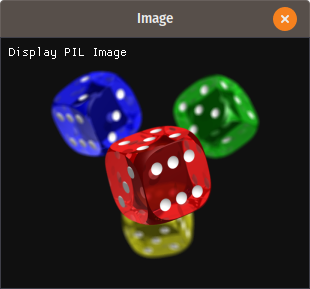 |
```python import bimpy from PIL import Image import numpy as np ctx = bimpy.Context() ctx.init(800, 800, "Image") image = np.asarray(Image.open("3.png"), dtype=np.uint8) im = bimpy.Image(image) while not ctx.should_close(): with ctx: bimpy.text("Display Image of type:") bimpy.same_line() bimpy.text(str(type(image))) bimpy.image(im) ``` | 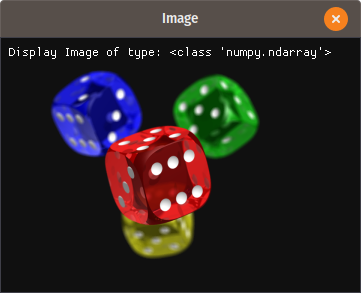 |
Core API | Using `bimpy.App` class |
```python import bimpy as bp ctx = bp.Context() ctx.init(600, 600, "Hello") s = bp.String() f = bp.Float() while not ctx.should_close(): with ctx: bp.text("Hello, world!") if bp.button("OK"): print(s.value) bp.input_text('string', str, 256) bp.slider_float("float", f, 0, 1) ``` | ```python import bimpy as bp class App(bp.App): def __init__(self): super(App, self).__init__(title='Test') self.s = bp.String() self.f = bp.Float() def on_update(self): bp.text("Hello, world!") if bp.button("OK"): print(self.s.value) bp.input_text('string', self.s, 256) bp.slider_float("float", self.f, 0, 1) app = App() app.run() ``` |
```python import bimpy from PIL import Image ctx = bimpy.Context() ctx.init(800, 800, "Image") image = Image.open("test.png") im = bimpy.Image(image) while not ctx.should_close(): with ctx: bimpy.text("Display PIL Image") bimpy.image(im) ``` | 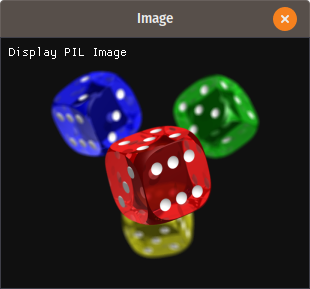 |
```python import bimpy from PIL import Image import numpy as np ctx = bimpy.Context() ctx.init(800, 800, "Image") image = np.asarray(Image.open("3.png"), dtype=np.uint8) im = bimpy.Image(image) while not ctx.should_close(): with ctx: bimpy.text("Display Image of type:") bimpy.same_line() bimpy.text(str(type(image))) bimpy.image(im) ``` | 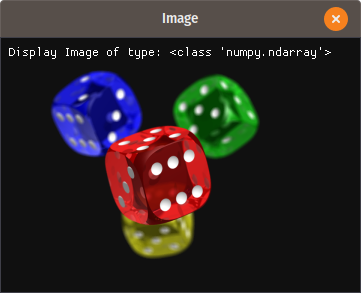 |